Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial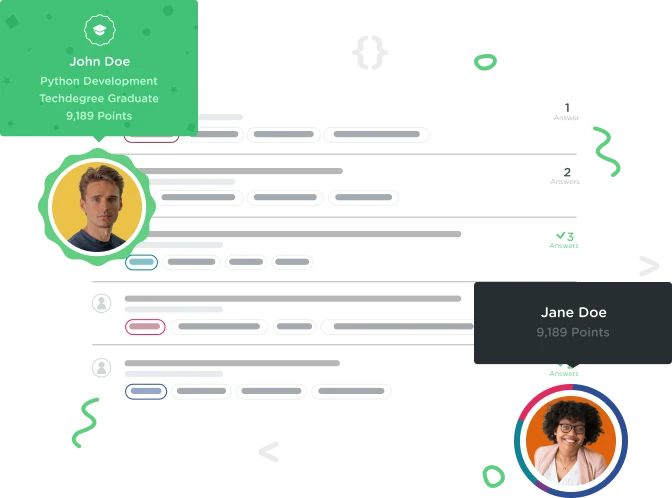

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 Pointsiterator
to get the first 4 and last 4 items in the iterable.Do we need to use 2 slices.?.
items = list(range(1,20))
def first_4(items):
return items[:4]
def odds(items):
return items[1::2]
3 Answers
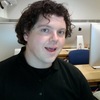
Nathan Smutz
9,492 PointsYou could delete the middle from item and return it; but this can be really bad form. Not to mention it requires more typing.
def first_and_last_4(item):
del item[4:-4]
return item
As I said, this is an example of a really bad idea. The original first_4 function copies the items out of the list and returns a new list. The function I listed above changes the original list that was passed in. If you use the list again after passing it to the function, the middle will be missing. If someone was counting on the original list staying the same, this would be a nasty bug. For such a thing, you'd want to make it clear in the function name that it modifies the input.
I think your best option is to use two slices:
def first_and_last_4(item):
return item[:4] + item[-4:]
Or, to be a little silly
def first_and_last_4(item):
return first_4(item) + item[-4:]
William Li
Courses Plus Student 26,868 PointsJust take the first 4 items and last 4 items from the list and put them together.
def first_and_last_4(items):
return items[:4] + items[-4:]

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsThank You very much. I don 't that in return we can append more one value.
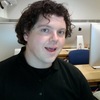
Nathan Smutz
9,492 PointsIn this case, Python added the two lists together before returning the new list. You can usually use "return" like you would use "=".
>>> new_list = [1, 2] + [3, 4]
>>> new_list
[1, 2, 3, 4]
>>>
>>>def func():
... return [1, 2] + [3, 4]
...
>>>func()
[1, 2, 3, 4]
>>>new_list == func()
True
SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsSUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsThank You very much for the detailed description and exploring different options. Nice.