Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial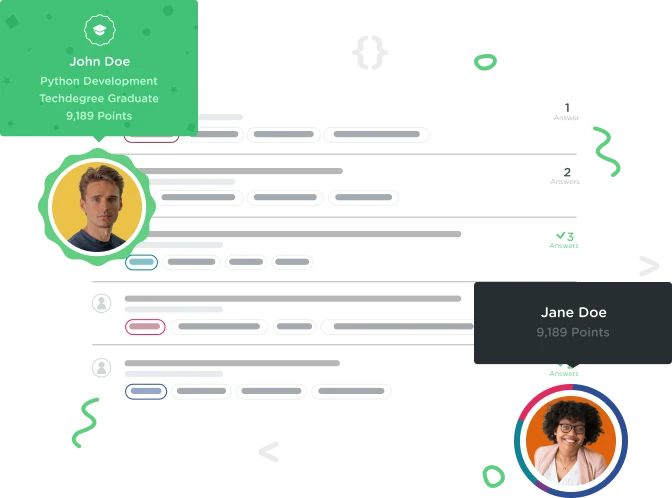

Tatenda Matambo
1,359 PointsIts c# objects inheritance The tasks is to come up with a code
The Polygon class represents a 2 dimensional shape. It has a public field named NumSides. Create a new type of polygon called Square. It should have a public readonly field named SideLength thatβs initialized using the constructor. Use base to initialize NumSides to 4.
1Polygon.cs namespace Treehouse.CodeChallenges 2 { 3 class Polygon 4 { 5 public readonly int NumSides; 6 β 7 public Polygon(int numSides) 8 { 9 NumSides = numSides; 10 } 11 } 12 }
1 Answer

Juan Naputi
1,772 PointsWe'll start off small and then work our way up.
The first thing that you have to do is make a class called Square and make it have a readonly field called SideLength that is initialized in the constructor. This provides all the information we need to create the class. Without worrying about deriving from the Polygon class, this is what we will have.
// Our basic, happy square.
class Square
{
public readonly int SideLength;
public Square(int sideLength)
{
SideLength = sideLength;
}
}
Now, we are told that it is a new type of Polygon. As such, we make our Square class derive (extend) from the Polygon class:
// Notice that we extended from Polygon.
// When you are extending a class, you put a ':' between your class name and the
// class you are extending.
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength)
{
SideLength = sideLength;
}
}
We are then told to use base to initialize NumSides to 4:
class Square
{
public readonly int SideLength;
// Notice the use of base here. We are calling the
// constructor of Polygon passing in the value of 4.
// This value will be assigned to the Polygons *NumSides* variable.
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
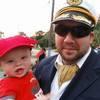
Christian Higgins
15,758 PointsThis is very thorough, Juan, thank you!!
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsWhat exactly is your question? Please be more specific.