Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial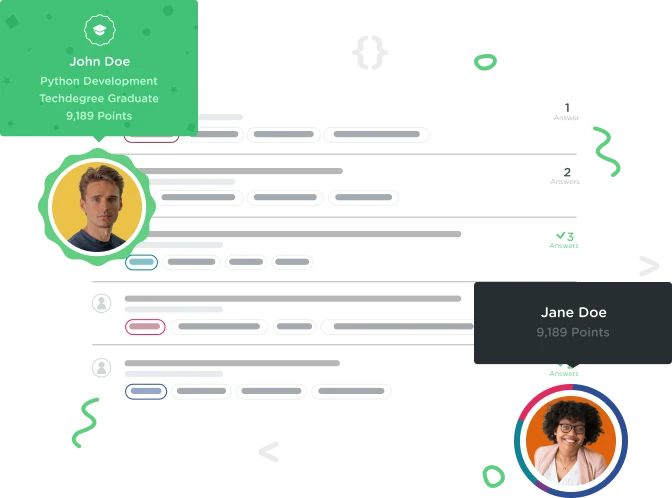
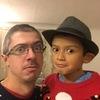
Matthew Goodman
12,786 PointsIt's Not Working?
var checkBox = taskListItem.querySelector("input[type=checkbox]");
on the JS console keeps saying :
Uncaught TypeError: Cannot read property 'querySelector' of undefined
4 Answers
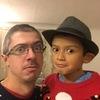
Matthew Goodman
12,786 PointsThanks for reply code as needed, cannot see what I have done all I know its a CSS selector but says its an error??. and how do I post code with the black background lol, many thanks
//Problem: user interaction does not provide results //Solution: Add interactivity so user can add daily tasks.
var taskInput = document.getElementById("new-task"); //new-task var addButton = document.getElementsByTagName("button")[0]; //first button var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//new task list item var createNewTaskElement = function(taskString) { //create list item var listItem = document.createElement("li");
//input (checkbox) var checkBox = document.createElement("input"); // checkBox //label var label = document.createElement("label"); //input(text) var editInput = document.createElement("input"); //text //button.edit var editButton = document.createElement("button"); //button.delete var deleteButton = document.createElement("button");
//each element needs modifying
//Each element needs appeneding
listItem.appendChild(checkBox); listItem.appendChild(label); listItem.appendChild(editInput); listItem.appendChild(editButton); listItem.appendChild(deleteButton);
return listItem; }
//Add a new task var addTask = function(){ console.log("add task..."); //Create a new list item with the text from new- task
var listItem = createNewTaskElement("some new task");
//append listItem to incompleteTaskHolder incompleteTasksHolder.appendChild(listItem);
}
//Edit an existing task var editTask = function() { console.log("edit task..."); //when the edit button is pressed // if the class of the parent is the .editmode //switch from edit mode //label text become the input value //else //switch to edit mode //input value becomes the labels text
//toggel edit mode on the parent } //Delete an existing task var deleteTask = function() { console.log("delete task..."); //when the delete button is pressed //remove the parent list item from the ul list }
//Mark a task as complete var taskCompleted = function() { console.log(" task complete..."); //when the check box is checked //append the task list item to the completed tasts }
//Mark a task as incomplete var taskIncomplete = function () { console.log("task incomplete..."); //when the check box is unchecked //we want to appent to the completed tasks
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) { console.log("Bind list item events"); //select its children var checkBox = taskListItem.querySelector("input[type=checkbox]"); var editButton = taskListItem.querySelector("button.edit"); var deleteButton = taskListItem.querySelector("button.delete");
//bind the edit task to edit button editButton.onclick = editTask;
//bind deleteTask to delete button deleteButton.onclick = deleteTask; //bind checkBoxEventHandler to the check box checkBox.onchange = checkBoxEventHandler; } //set the click handler to the addTask function addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items for(var i = 0; i < incompleteTasksHolder.children.length; i++);{
//bind events to list item children(taskcompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted); }
//cycle over completeTaskHolder ul list items for(var i = 0; i < completedTasksHolder.children.length; i++);{ //for each li item //bind events to list item children(taskIncompleted) bindTaskEvents(completedTasksHolder.children[i], taskIncompleted); }
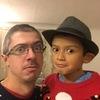
Matthew Goodman
12,786 Points//Proble: user interaction does not provide results
//Solution: Add interavtivity so user can add daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//new task list item
var createNewTaskElement = function(taskString) {
//create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); // checkBox
//label
var label = document.createElement("label");
//input(text)
var editInput = document.createElement("input"); //text
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//each element needs modifying
//Each element needs appeneding
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function(){
console.log("add task...");
//Create a new list item with the text from new- task
var listItem = createNewTaskElement("some new task");
//append listItem to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
}
//Edit an existing task
var editTask = function() {
console.log("edit task...");
//when the edit button is pressed
// if the class of the parent is the .editmode
//switch from edit mode
//label text become the input value
//else
//switch to edit mode
//input value becomes the labels text
//toggel edit mode on the parent
}
//Delete an existing task
var deleteTask = function() {
console.log("delete task...");
//when the delete button is pressed
//remove the parent list item from the ul list
}
//Mark a task as complete
var taskCompleted = function() {
console.log(" task complete...");
//append the task list item to the completed tasts
var listItem = this.parentNode;
completedTasksHolder.appendChild(this.parentNode);
}
//Mark a task as incomplete
var taskIncomplete = function () {
console.log("task incomplete...");
//when the check box is unchecked
//we want to appent to the completed tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select its children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind the edit task to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to the check box
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++);{
//bind events to list item children(taskcompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completeTaskHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++);{
//for each li item
//bind events to list item children(taskIncompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncompleted);
}
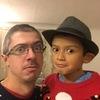
Matthew Goodman
12,786 Pointsthis is what the console says??
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select its children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
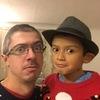
Matthew Goodman
12,786 Pointssorry that did not show
Rich Donnellan
Treehouse Moderator 27,696 PointsRich Donnellan
Treehouse Moderator 27,696 PointsPost the rest of your code using Markdown.