Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial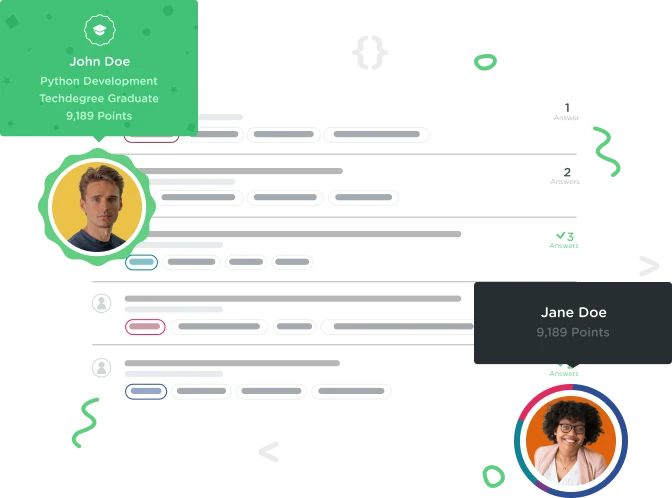

gladys padilla
7,909 PointsIt's official: you're awesome at Python dictionaries! One last task and then you can take a well-deserved break!
I've done this so many ways, I do not know what else I'm doing wrong! I've done arguments, loops, have done append with different lists, I just don't know anymore. Someone please help!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teachers):
count = 0
for teachers in teachers:
count += 1
return count
def num_courses(arg):
answer = 0
for key, value in arg.items():
answer += len(value)
return answer
def courses(teachers):
course_list = []
for value in teachers.values():
course_list += value
return course_list
def most_courses(teacher_dict):
max_count = ['name', 0]
for key in teacher_dict:
if len(teacher_dict[key]) > max_count[1]:
max_count[0] = key
max_count[1] = len(teacher_dict[key])
return max_count[0]
def stats(teachers):
result = []
for teacher, courses in teachers.items():
stats = [key, len(courses)]
result.append(stats)
return result
2 Answers
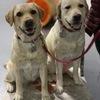
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Gladys,
You have a minor typo in your stats
function.
In this line: stats = [key, len(courses)]
you refer to key
but you've not defined a variable called key
. I think you mean teacher
.
Hope that helps
Cheers
Alex
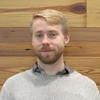
Cody Selman
7,643 PointsHello Gladys,
I don't want to just give you an answer outright, as there's no fun in that. The suggestion I'd like to make and that I hope will send you on the correct path is to redo your for loop. I'm not familiar with the syntax you're using, but it seems a bit overly complex (and possibly incorrect? I'm new to Python myself, so i can't say for sure but VS Code gives me an error when i paste that into my editor).
Please consider this example:
for teacher in teachers:
# This will print the teacher's name
print (teacher)
# This will print the array of courses
print (teachers[teacher])
Accessing the data in that manner will steer you in the right direction, I hope! If that doesn't help at all and you're still stuck, just comment here and I'll elaborate further.
Best regards,
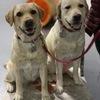
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Cody,
The .items()
dictionary method is a really convenient way of iterating through a dictionary and getting access to both the key and value at the same time.
In the way that Gladys has structured it, for teacher, courses in teachers.items():
, she is iterating through the teachers
dictionary, and on each iteration she gets both the key (in the variable teacher
) and the value (in the variable courses
). You'll often see people make this explicit, calling those temporary variables key
or k
and value
or v
(like in the example from the official docs I linked).
So you could do this:
for key, value in teachers.items():
# this will print the teacher's name
print(key)
# this will print the array of courses
print(value)
This is a really handy technique and you'll start seeing it all the time on your Python journey!
Happy coding,
Alex