Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial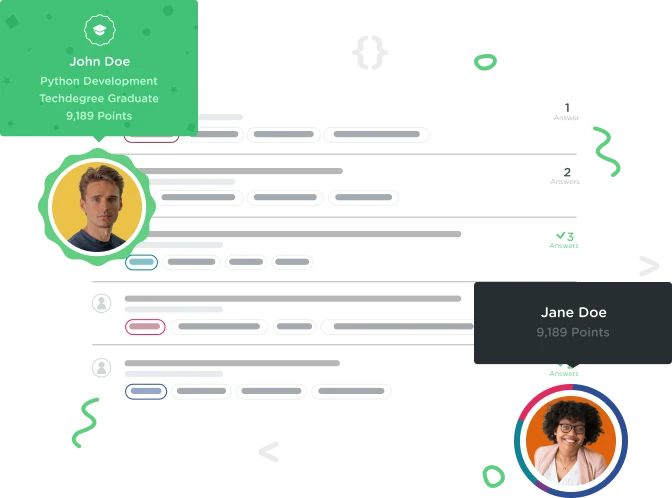
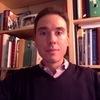
Giovanni Rinaldi
1,104 PointsIt's very hard understand instance
I have problems with initializers. Could someone help me? Thanks a lot
Giovanni
// Enter your code below
class Shape {
var numberOfSides: Int
let someShape: Shape
init(numberOfSides: Int, someShape: Shape) {
self.someShape = Shape(numberOfSides: Int, someShape: Shape)
}
}
3 Answers
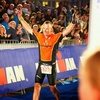
Steve Hunter
57,712 PointsHi Giovanni.
You're pretty much there - just with a couple of issues in your class. First, let's worry about the someShape
thing later. That's what we do after we've completed the class definition.
So, we want a clas with one stored property, a variable called numberOfSides
. You've done that just fine already; it looks like this:
class Shape {
var numberOfSides: Int
}
But we need an initialiser method. The purpose of init
is to set the stored properties, in this case, just numberOfSides
. Nothing else needs to happen inside init
. It looks like:
init(numberOfSides: Int){
self.numberOfSides = numberOfSides
}
So, we pass in a number and the init
method assigns it to self.numberOfSides
. That's the class defined. Now for the instance that the challenge asks for. This must be outside the class so write a line underneath the closing braces. You want to pass in numberOfSides
too. This looks like:
let someShape = Shape(numberOfSides: 3)
And that's it - I hope that helps.
Steve.
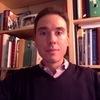
Giovanni Rinaldi
1,104 PointsThanks, Steve!
You're great and your explanation helps me a lot. I'm totally beginner and I'm just thinking that I will study Object-Oriented lessons for a lot. Have a good day
Giovanni
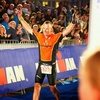
Steve Hunter
57,712 PointsStudying OOP is a good foundation. It is common across many languages so the concepts are totally transferable between different languages.
Good luck and shout if you need help!
Steve.
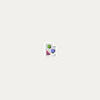
doesitmatter
12,885 PointsA class is like a blueprint for something. Say you want to build a car, you create a blueprint for that car, you give that blueprint to a factory and the factory makes a car object, the car object then is an instance of the car class.
class Car{
//variable properties or attributes
var numberOfWheels
var color
//initialization method, setting the values for the values and attributes, which arent initialized
init(color : String, numberOfWheels: Int){
self.color = color
self.numberOfWheels = numberOfWheels
}
//methods, things which a Car can do
startCar(){
print("Vroom vroom!")
}
//etc...
}
Now we can easily create a car with:
let someCar = Car(color : "Red", numberOfWheels: 4);
let someCar = Car(color : "Green", numberOfWheels: 8);
let someCar = Car(color : "Blue", numberOfWheels: 6);
Suppose we didn't have the init method, then it would be useless blueprint, because it would only be able to make one type of car!
class Car{
//variable properties or attributes
var numberOfWheels = 4
var color = "Red"
//methods, things which a Car can do
startCar(){
print("Vroom vroom!")
}
//etc...
}
let redCarWithFourWheels = Car();