Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial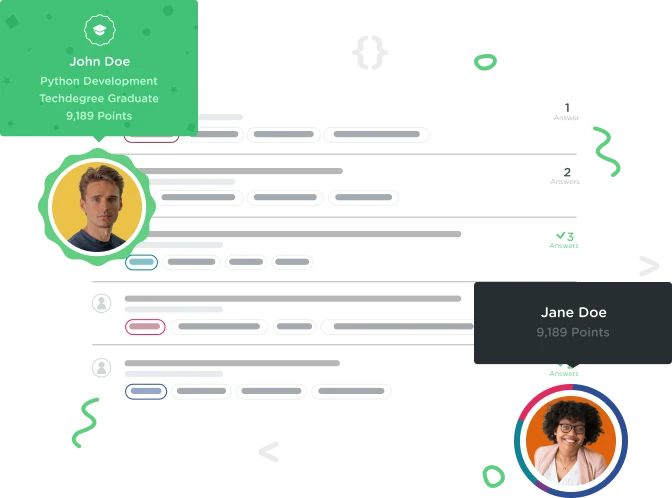

Thomas Katalenas
11,033 PointsI've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet th
it says I can't do fieldName[0]
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if(fieldName[0] != 'm'){
continue;
}else{throw IllegalArgumentException;}
return fieldName;
}
}
3 Answers
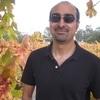
Kourosh Raeen
23,733 PointsTry the charAt() method. Call it on fieldName and pass 0 to it as the index.

Thomas Katalenas
11,033 Pointspublic class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if( fieldName.charAt(0) == 'm')
continue;
if (fieldName.charAt(1) == fieldName.charAt(1).toUpperCase())
return fieldName;
}
}
./TeacherAssistant.java:9: error: continue outside of loop
continue;
^
./TeacherAssistant.java:10: error: char cannot be dereferenced
if (fieldName.charAt(1) == fieldName.charAt(1).toUpperCase())
^
2 errors
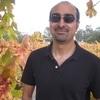
Kourosh Raeen
23,733 PointsThe continue keyword is used in loops. You need an if-else statement. In the if part check to see if fieldName doesn't start with 'm' or the second letter of fieldName is not uppercase. If that's the case you need to throw an IllegalArgumentException exception. In the else part return fieldName.
Also, you can make use of the isUpperCase() method of the Character class to see if the second letter is uppercase:
!Character.isUpperCase(fieldName.charAt(1))