Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial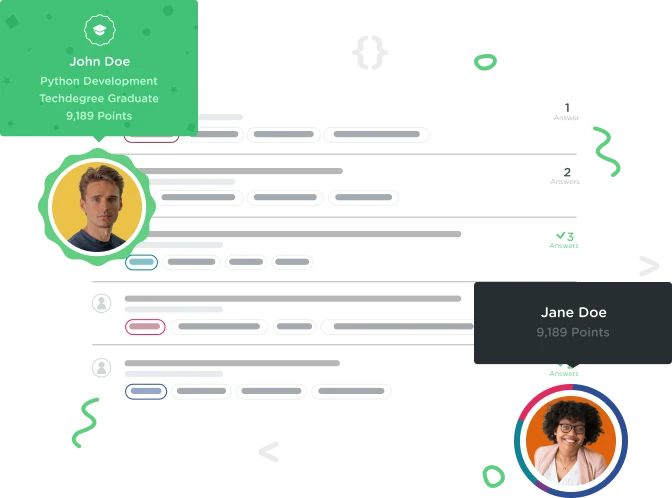

Thomas Katalenas
11,033 PointsI've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet th
Here are the errors!!
./TeacherAssistant.java:8: error: char cannot be dereferenced
if(fieldName.charAt(0) == 'm' && fieldName.charAt(1) == fieldName.charAt(1).toUpperCase()){
^
./TeacherAssistant.java:10: error: cannot find symbol
}else{throw IllegalArgumentException("first letter doesn't start with m or second letter not Uppercased");
^
symbol: method IllegalArgumentException(String)
location: class TeacherAssistant
2 errors
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if(fieldName.charAt(0) == 'm' && fieldName.charAt(1) == fieldName.charAt(1).toUpperCase()){
return fieldName;
}else{throw IllegalArgumentException("first letter doesn't start with m or second letter not Uppercased");
}
}
}
3 Answers
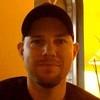
Jeremy Hill
29,567 PointsI would do it this way:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if(fieldName.charAt(0) != 'm' || Character.isUpperCase(fieldName.charAt(1)) == false)
throw new IllegalArgumentException("Not a valid Field Name!");
else
return fieldName;
}
}

Thomas Katalenas
11,033 Pointswhere are you getting Character from ?
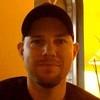
Jeremy Hill
29,567 PointsIf you are familiar with java.lang.Object, it is a class that ALL objects inherit from; well the Character class is a child class of java.lang.Object as well. To put it in easier terms, all Objects have access to the class Character and all of its methods. so the method isUpperCase() as well as isLowerCase() both are in the Character class. In order to use the methods just type Character. and then the method that you are wanting to use. If you want to know more about it go this website and try using some of the methods from it- you will find them really helpful once you know about them.
http://docs.oracle.com/javase/7/docs/api/java/lang/Character.html
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsYou should be using the '||' (or) character instead of the && (and) because when you use "and" you are saying that both conditions have to be met in order to throw the exception; in this case it just needs to be one or the other.