Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial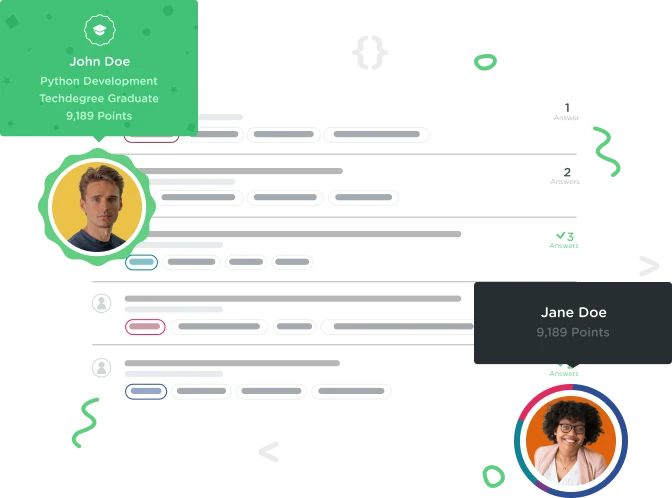

Tojo Alex
Courses Plus Student 13,331 Pointsi've already declared a method named SetNumFliesEaten
I already declared the method so why does it give me the error that I didn't declare it.
namespace Treehouse.CodeChallenges
{
public class Frog
{
private int _numFliesEaten;
public int GetNumFliesEaten
{
get
{
return _numFliesEaten;
}
}
public int SetNumFliesEaten
{
set
{
_numFliesEaten = value;
}
}
}
}
8 Answers
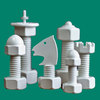
Steven Parker
230,274 PointsDid you peek ahead at the next lesson? It looks like you're mixing method syntax with property syntax.
For this challenge, just create simple methods. The definitions must have parentheses, and the setter method will require an argument.

Tojo Alex
Courses Plus Student 13,331 Pointsi have watched the video though I don't see how i converted the syntax to property syntax
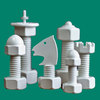
Steven Parker
230,274 PointsProperty declarations don't have parentheses, and inside them you have accessors with the names "get" and "set". So the code shown above appears to have two partially-implemented properties.
On the other hand, accessor method declarations would have parentheses (with or without arguments) after their names, and the body would contain the code itself.

Tojo Alex
Courses Plus Student 13,331 Pointsyes I know that though why would it ask me to write the setnumflies method again if it's clear that i already done that
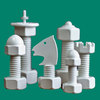
Steven Parker
230,274 PointsWhat you have here is a "SetNumFliesEaten" property, but the instructions ask for a "SetNumFliesEaten" method.

Tojo Alex
Courses Plus Student 13,331 Pointsjust to check is the first method in property form
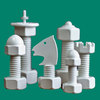
Steven Parker
230,274 PointsThere are no methods in the code shown above, but two incomplete properties. Method definitions would have parentheses.

Tojo Alex
Courses Plus Student 13,331 Pointsjust to see, could you type out the correct parentheses for the method syntax so I don't mix up with property syntax, parentheses is the only thing that confuses me on the keyboard
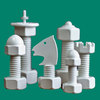
Steven Parker
230,274 PointsA generic "getter" method might look like this:
public int GetSomeLocalValue() // <-- methods have parentheses, even if empty
{
return _someLocalValue;
}

Tojo Alex
Courses Plus Student 13,331 Pointsok thanks it makes life easier for me :D
I don't understand why it gives the error:
Frog.cs(10,12): error CS1525: Unexpected symbol {'
Frog.cs(18,12): error CS1525: Unexpected symbol
{'
Compilation failed: 2 error(s), 0 warnings
''' namespace Treehouse.CodeChallenges { public class Frog { private int _numFliesEaten;
public int GetNumFliesEaten()
{
get
{
return _numFliesEaten;
}
}
public int SetNumFliesEaten()
{
set
{
_numFliesEaten = value;
}
}
} } '''
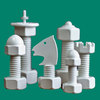
Steven Parker
230,274 PointsTake a closer look at the sample getter method I wrote, you'll see it contains code directly, with no inner "get" block. That's also something only used on properties. Also:
- setter methods need to take an argument
- setter methods normally don't return anything (return type "void")

Tojo Alex
Courses Plus Student 13,331 Pointsi have removed the return syntax and the getter method though it gives me the error : Frog.cs(18,9): error CS1525: Unexpected symbol `{' Compilation failed: 1 error(s), 0 warnings
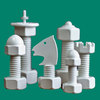
Steven Parker
230,274 PointsYou must be pretty close by now. Try exercising your debugging skills. An unexpected symbol is often caused by something missing that should be in front of it.
This thread seems to have gone far afield of the original question. If you give it your best shot and still haven't resolved it, please close out this question and start a new one showing what your current code looks like there.