Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial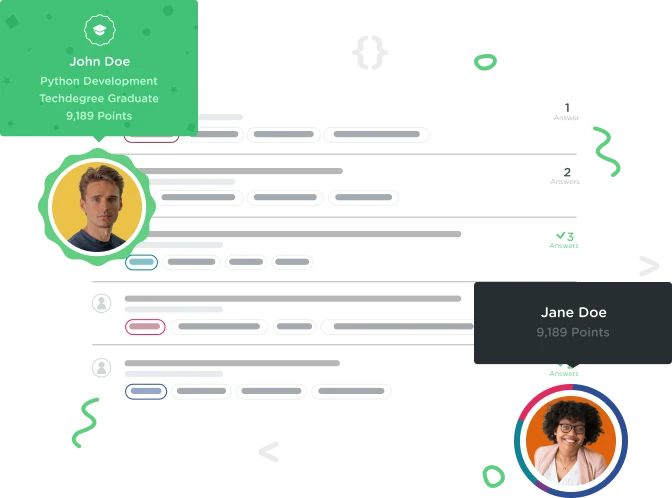
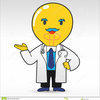
Cameron Hastings
3,439 PointsI've been at this for four hours.
If someone could just provide the solutions to task 4/4, I'll be your best friend.
Seriously, I've been at this all day. I accept defeat on this one.
The problem lies within Main.java
"So close now! Okay so now un-comment the comments in Main.java and Forum.java. PS. You're awesome!"
"After you uncomment the code in Main.java and Forum.java, fix the code as described in the comments of Main.java. The code in main is attempting to use the code you just fixed."
public class Forum {
private String topic;
public Forum (String topic) {
this.topic = new topic();
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = new firstName();
this.lastName = new lastName();
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost(User author, String title, String description) {
this.author = new author();
this.title = new title();
this.description = new description();
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0], args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "asdf", "ASDF");
forum.addPost(post);
}
}
2 Answers

Samuel Ferree
31,722 PointsYour problem lies in your class constructors for User, Forum, and ForumPost. You want to assign the private fields, to the parameters passed into the constructor. Instead you are calling the passed in parameters as constructors for classes that don't exist.
For instance, in User.java
public User(String firstName, String lastName)
{
// instead of this.firstName = new firstName()
this.firstName = firstName;
this.lastName = lastName;
}

Maurice Abney
Courses Plus Student 9,859 Pointsit's looks like your problem lies in your forum.java, user.java, and forumpost.java files. Specifically in your constructors. You arent using them properly. The appropriate format would be
public Forum (String topic) {
this.topic = topic;
}
and the same for the other constructors. Your'e currently using the new keyword when you don't need to.
Cameron Hastings
3,439 PointsCameron Hastings
3,439 PointsIt worked.... thank you!
Richard Merritt
962 PointsRichard Merritt
962 PointsCan you go into more detail with this?