Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial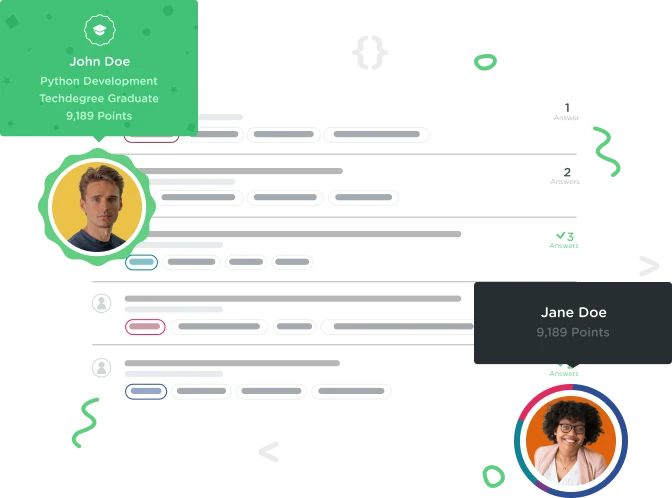

lee lewis
Courses Plus Student 5,796 Pointsive been stuck on this problem for three days and nobody can help... what do i do????
Bummer! Hmmm, I entered "Nelson" for the lastName and expected line number 2 to be returned, but instead it was 0
whats going on???????
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name*/
char getLetter = lastName.charAt(0);
/* Line 1 - A thru M*/
if (getLetter >= 'a' && getLetter <= 'm') {
lineNumber = 1;
}
if (getLetter >= 'n' && getLetter <= 'z') {
lineNumber = 2;
}
/* Line 2 - N thru Z*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer

Ian Billings
Courses Plus Student 7,494 PointsHi,
I think the problem is you are passing a lastName String that has a capital letter character at the beginning and then in the method you are comparing that to lower case characters. So I would change the second line of the getLineNumberFor method to:
char getLetter = lastName.toLowerCase().charAt(0);
Moira Lawrie-Martyn
8,073 PointsMoira Lawrie-Martyn
8,073 PointsI agree with Ian on this one. Remember, A and a are at two different positions in the ASCII table.
'A' is at decimal position 65, and 'a' is at decimal position 97, so the code is trying to match 97 and ignoring 65 because it doesn't match.
If you change it to lower case as Ian has done, or you change the search parameter to 'A', it should fix the problem.