Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial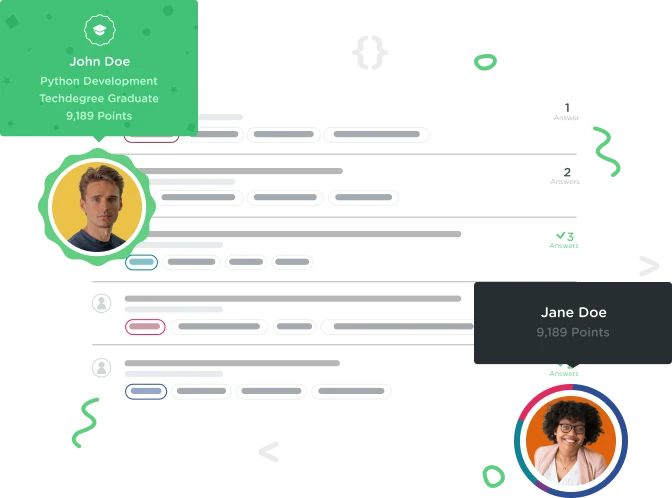
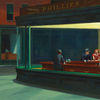
Anthony Grodowski
4,902 PointsI've got a problem!
I stilll don't get that whole self thing... Also how can i use kwargs in my code? I get that kwargs is useful in creating a new instance in the REPL but how could it be in the main code? I'm really confused and be so grateful for any help!
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, laps = RaceCar.laps, **kwargs):
self.fuel_remaining = fuel_remaining
self.color = color
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.fuel_remaining -= length * 0.125
RaceCar.laps += 1
1 Answer
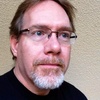
Chris Freeman
Treehouse Moderator 68,423 PointsGood question! When defining a class method you are required to provide a parameter that will be used to hold a reference to the class instance. This self
parameter gets assigned to a value (the instance id. It’s location in memory) when the class instance is created. That is why you don’t need to provide the self
parameter when calling class methods. Since self
points to the instance, all the attributes can be referenced used the “dot” notation of self.attribute
.
Each object has a Namespace dict that holds its attributes, name of methods, etc. The class RaceCar
has its own. Each instance of the class also has its own NameSpace of attributes, etc. When looking up a reference, the local instance NameSpace is checked first, if not found then the class NameSpace is checked.
When setting any attribute using self.attribute = value
, the attribute immediately becomes entered into the instance NameSpace thus becoming a local variable to the class instance. The class attribute laps
becomes overridden (or in a sense hidden) from the instance because the local laps
is found first.
The **kwargs is used to receive an unknown number of keyword arguments. The variable kwargs
is referenced to unpack the key/value pairs when needed.
Using RaceCar.laps
refers to the class attribute laps
. It can’t be referenced inside of RaceCar
because it hasn’t finished being defined yet. It doesn’t yet exist.
There are some errors in your code:
- the
__init__
parameterlaps
needs to set to a default value of 0, as inlaps=0
- the instance attribute
self.laps
must be assigned to the argument passed intolaps
. This is done the same way asself.color
is assigned - in
RaceCar.run_lap()
, it is the instance attributeself.laps
that needs to be set.RaceCar.laps
is not yet defined
Edits: fixed typo self,attribute
should be self.attribute
Expanded answer.
Post back if you need more help. Good luck!!!
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsThank you, that helped! I still have some problems in understanding self - "The self parameter gets assigned to a value (the instance I’d) when the class instance is created. That is why you don’t need to provide the self parameter when calling class methods." - could you please explain it in another words? Also "When setting any attribute using self,attribute =, the attribute immediately becomes a local variable to the class instance. The class attribute laps becomes overridden or in a sense hidden from the instance." is a little bit unclear for me... Thanks in advance!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsI’ve expanded my answer above.
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsAlright, things are going great except for that cursed self hah. Sorry to bother you, but I think I still don't understand self usage and meaning of it - could you please bring in some examples with explanation? From the basics if possible.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsIt’s not too important to understand everything about
self
immediately. It may be enough to know:@classmethod
)self
refers to the current instanceself.attribute_name