Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial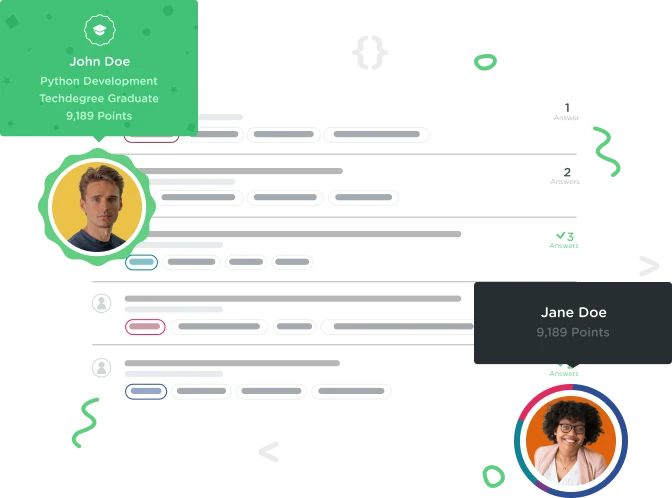

Maksim Nesterenko
1,387 PointsI've got a TypeError instead of printing out message to the console
Here is my code
var http = require('https');
var username = 'maksimnesterenko123';
var url = 'https://teamtreehouse.com/' + username + '.json';
//Print out message
function printMessage(userName, badgeCount, points) {
var message = userName + ' has ' + badgeCount + ' total badge(s) and ' + points + ' points in JavaScript';
console.log(message);
}
//Print out error messages
function printError(error) {
console.error(error.message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get(url, function(response) {
var body = "";
//Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if(response.statusCode === 200) {
try {
var profile = JSON.parse(body);
printMessage(username,profile.badges.length, profile.points.JavaScript);
} catch(error) {
//Parse Error
printError(error);
}
} else {
//Status Code Error
printError({message: "There was an error getting a profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"});
}
});
//Parse the data
//Print the data
});
//Connection error
request.on('error', printError);
And an error in the console:
/home/treehouse/workspace/app.js:38
error getting a profile for " + username + ". (" + http.STATUS_CODES[response.
^
TypeError: Cannot read property '404' of undefined
at IncomingMessage.<anonymous> (/home/treehouse/workspace/app.js:38:110)
at IncomingMessage.emit (events.js:129:20)
at _stream_readable.js:908:16
at process._tickCallback (node.js:355:11)
Please help me find a mistake
(Edited by BothXP: to add markdown code formatting.)
2 Answers
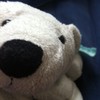
bothxp
16,510 PointsHi,
The 'TypeError: Cannot read property '404' of undefined' is a good hint as to what is going on.
In your code you use :
var username = 'maksimnesterenko123';
var url = 'https://teamtreehouse.com/' + username + '.json';
But trying to open https://teamtreehouse.com/maksimnesterenko123.json returns a HTTP 404 'not found' message.
Does the user maksimnesterenko123 exist ? Try just removing the 123 from the end of the username and I think that it should all work.
---- Ok I'm being dumb aren't I ! The whole point of this part of the course is to generate the correct error message :-/ I'll leave this here for your amusement whilst I pop off to have a look at what the expected result was supposed to be ----
Right ... I'm back!
This appears to be to do with the move from http to https mentioned in the teachers notes of an earlier video. When I first did this course it made use of http, not https. Updated code:
var https = require('https');
var http = require('http');
var username = 'maksimnesterenko123';
var url = 'https://teamtreehouse.com/' + username + '.json';
//Print out message
function printMessage(userName, badgeCount, points) {
var message = userName + ' has ' + badgeCount + ' total badge(s) and ' + points + ' points in JavaScript';
console.log(message);
}
//Print out error messages
function printError(error) {
console.error(error.message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get(url, function(response) {
var body = "";
//Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if(response.statusCode === 200) {
try {
var profile = JSON.parse(body);
printMessage(username,profile.badges.length, profile.points.JavaScript);
} catch(error) {
//Parse Error
printError(error);
}
} else {
//Status Code Error
printError({message: "There was an error getting a profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"});
}
});
//Parse the data
//Print the data
});
//Connection error
request.on('error', printError);
Now seems to work. Changes made:
I changed the opening of the code to require both http & https.
var https = require('https');
var http = require('http');
I then changed line 19 to make use of https
var request = https.get(url, function(response) {
and I think that was all that I did.
The issue is that http.STATUS_CODES is used in the code to provide a collection of all the standard HTTP response status codes, and the short description of each. But https.STATUS_CODES does not exist. You are going to need to var https = require('https');
and var http = require('http');
so that you can access both the https://teamtreehouse.com/ json feed as well as access the http.STATUS_CODES.
Does that make sense?
Hey Andrew Chalkley I noticed that this issue seems to have come up a few times now since the url changed over the using https. I saw the teachers note on https://teamtreehouse.com/library/nodejs-basics/building-a-command-line-application/making-a-get-request-with-http but I wonder if it would be worth adding a note to this video about the STATUS_CODES issue? Thanks

Maksim Nesterenko
1,387 PointsThank you for your help!
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherGood point!