Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial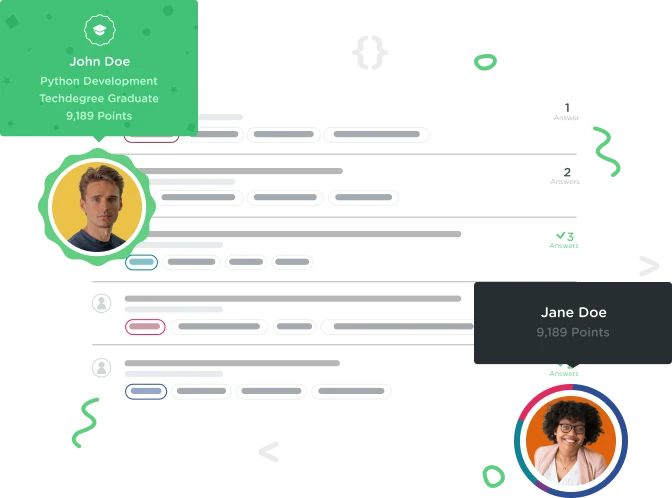

Moses Ong
889 PointsI've no idea how to print "Hi" as many times as the count statement.
This is my solution:
def printer(count): count = int(count) * ("Hi ") print("Hi ")
printer(3)
def printer(count):
print("Hi ")
printer(5)
2 Answers
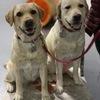
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Moses,
You've figured out the central issue to this problem, that we want to multiply count by the string we want to repeat. However, it looks like you're a bit confused about how we work with variable assignment and printing. Let's take a look at this line:
count = int(count) * ("Hi ")
Here you are taking the value passed into the function, attempting to convert it to an int, then multiplying it with 'Hi' to create a string that is the 'Hi' repeated count
number of times. This is exactly what we want to output to the user, but instead of doing that, you are taking this and replacing the count variable with your output string.
That's not necessarily wrong (you could print your new count variable in the subsequent line and pass the challenge), but it is kind of misleading. You'd always expect a variable named 'count' to contain a number, not a string.
If you really wanted to put the output value into a variable before printing it (not necessary in this challenge but in the real world you might want to do something else with that value, not just print it) then it would be better to pick a variable name that described the kind of value that it contains. Maybe 'output_string' or 'repeated_greetings'.
Now let's look at your last line:
print("Hi")
It's pretty obvious when we look at this line in isolation that the instruction is only going to print "Hi" and not the repeated his that you wanted. When you think about it, there's no way for this print function to know that you want to output your repeated value. So the solution is either to pass into print the variable that you updated in the previous line, or to put the construction of your repeated his into the print function. For example, suppose we had "hello " and "world" and we wanted to combine them for printing. We could either do this:
combined = "hello " + "world"
print(combined)
Or, since the manipulation we are doing is quite simple, it's clear and easy to read if we just put the combining into the call to the print function:
print("hello " + "world")
Hope this clears everything up for you,
Cheers
Alex

Moses Ong
889 PointsHey Alex,
Thanks for the prompt reply,
Here's my updated code and it works
def input(count): count = int(count) combined = count * "Hi " return combined
avg = input(10) print(avg)
I'm not sure this is suppose to be done this way, but I was able to get the answer from it :)