Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial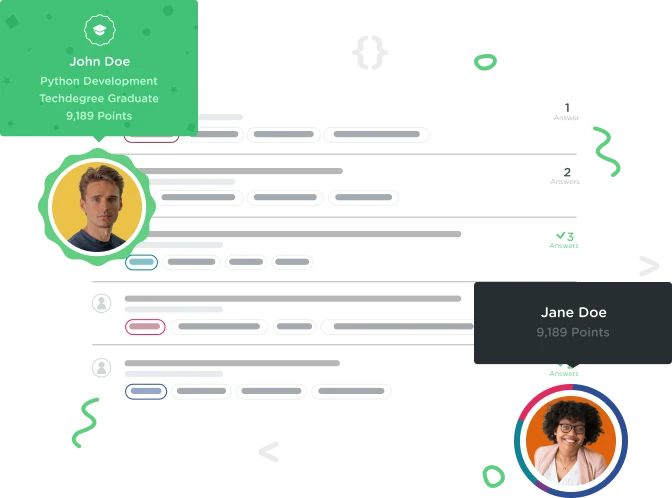
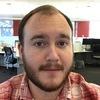
Amos Baynes
2,294 PointsI've tried a few variations on this problem, but I keep getting this error: TypeError: can't multiply sequence by non-in
I thought the except TypeError: was supposed to catch this error, but it's still throwing it.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
int(num)
except TypeError:
return num * len(num)
else:
return num * num
2 Answers
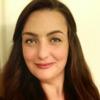
Jennifer Nordell
Treehouse TeacherHi there! The type error is actually being produced from your else
clause. Remember, the int
method to convert to an integer can accept either a number or a string. You should be checking for a ValueError
here.
In your try
part, you have int(num)
. Imagine for a minute that we send in "27" as a string. That is a string that can be converted to an integer, and thus neither the TypeError
nor the ValueError
will be triggered and it will hit the else
clause. At that point, you then try to multiply the string 27 by the string 27 which can't be done. This is where you're getting the TypeError
from. To fix this, in your try block you need to overwrite the current value of num
with the num
after it's converted to an integer. That way, when your else clause is hit, it will be an integer times an integer.
Hope this helps, but let me know if you're still stuck!
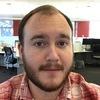
Amos Baynes
2,294 PointsGot it! Thanks a lot for your help!
Amos Baynes
2,294 PointsAmos Baynes
2,294 PointsThanks for the quick reply, Jennifer. I'm not clear what you mean by "overwrite the current value of num with the num after it's converted to an integer."
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi again! A quick hint:
num = int(num)
.Again,
int
can take either a string or a number. The string "42" can be converted to an integer. The string "Treehouse is awesome" can not be converted to an integer. And this is where we get aValueError
. The type is correct (a string or integer), but the value is such that it still can't be done.So assuming we sent in the value "42" as a string, you're trying the
int("42")
essentially. But the results of that aren't saved anywhere if it was successful. At this point, the value ofnum
will still be the string "42". The line I gave above says take whatevernum
was before and overwrite it with the integer version of that string if possible.