Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial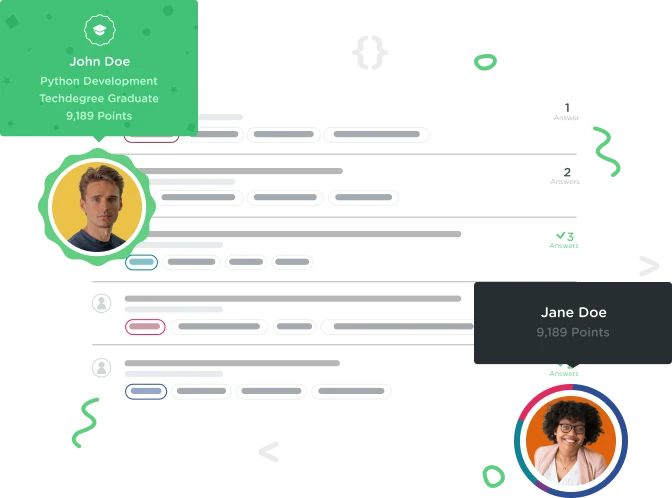

Johnny Harrison
4,331 PointsI've tried everything. I can't find the bug
Snapshot to my workspace: http://w.trhou.se/uigt3ey2pc
When I run this program, it will spit out the correct result only if every answer is true(TTT), or every answer is false(FFF). If I answer in any other combination (FTT), (TFF), (TTF) etc, some of the values will print to the screen, and others will come back as undefined.
Here's the loop that runs through every question:
for ( var i = 0; questions[0].length>=i; i++) {
guesses.push(prompt(questions[i][0]));
if (guesses[i] === questions[i][1]) {
correct.push(questions[i][0]);
correctList += "<li>" + correct[i] + "</li>";
counter++;
} else if (guesses[i] !== questions[i][1]) {
incorrect.push(questions[i][0]);
incorrectList += "<li>" + incorrect[i] + "</li>";
}
}
And here are some console tests I ran after answering the quiz (TFF):
>correct
["What is 5 + 8?"]
>correctList
"<ol><li>What is 5 + 8?</li></ol>"
//good, that's working as intended
>incorrect
(2) ["The primary colors are red, yellow and:", "Hot is to red as cold is to:"]
//looking good
>incorrectList
"<ol><li>undefined</li><li>undefined</li></ol>"
//What?!
the problem seems to be with passing them into the 'incorrectList' string.
1 Answer
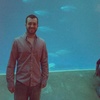
Kyle Johnson
33,528 PointsThe reason your list items are undefined is because the correctList or incorrectList is referencing the wrong position[i] in the correct or incorrect arrays. You don't want to know what the array values are for 0, 1 and 2 if let's say the correct array has 0 and the incorrect array has 0, 1.
You need to remove [i]
from
correctList += "<li>" + correct[i] + "</li>";
and
incorrectList += "<li>" + incorrect[i] + "</li>";
Kyle Johnson
33,528 PointsKyle Johnson
33,528 PointsYou could actually refactor your code like this too:
Johnny Harrison
4,331 PointsJohnny Harrison
4,331 PointsThanks for your help Kyle!