Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial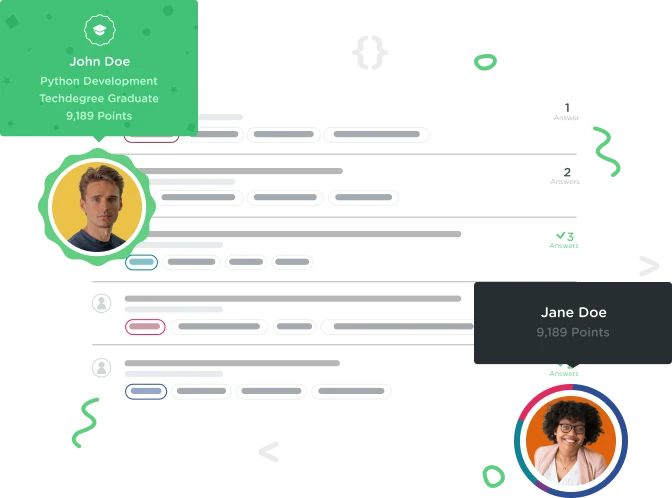

Michael Criste
5,045 PointsI've tried various approaches but without success. It's a syntax error that I need help with.
I understand the concepts just fine. I tried using "" in place of string. I tried Fixnum in place of number. I tried [] in place of array. I'm pretty sure I tried the variables with quotes around them, like in the question: 'string,' 'number,' etc.
Help!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter (array) {
if (typeof array === string){
return 0;
}
elsif (typeof array === number) {
return 0;
}
elsif (typeof array === 'undefined') {
return 0;
}
else
return array.length;
}
</script>
</body>
</html>
3 Answers
William Li
Courses Plus Student 26,868 PointsHi, a few things need to be fixed
- no
elsif
keyword in JavaScript, useelse if
. -
"string"
and"number"
instead of juststring
,number
when checking for equality with the output of typeof
function arrayCounter(array) {
if (typeof(array) === "string"){
return 0;
}
else if (typeof(array) === "number") {
return 0;
}
else if (typeof(array) === 'undefined') {
return 0;
}
else {
return array.length;
}
}

Richard Ling
8,522 PointsHi Michael,
You have used "elsif", instead of "else if", so:
function arrayCounter (array) {
if (typeof array === string) {
return 0;
} else if (typeof array === number) {
return 0;
} else if (typeof array === 'undefined') {
return 0;
}
else
return array.length;
}
Hope this helps :)
Thanks, Rich

Ian Hattendorf
7,715 PointsHi Michael, you have two issues with your code. First, you need to use else if
instead of elsif
. Second, typeof
returns a string, so you need to compare typeof array
to 'string'
instead of string
, same with number
.