Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial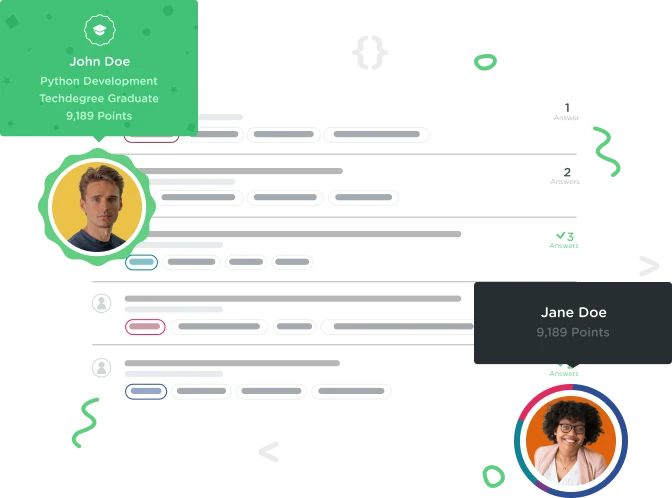

Jason Laux
3,128 PointsI've uncommented line of code, but still doesn't pass.
I get this error when I use the following code from the challenge.
Bummer! Did you uncomment the line in Example.java? The output from System.out does not show the 1 Yoda PEZ dispenser.
In Example.java, I've uncommented the last line:
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
cart.addItem(dispenser);
}
}
And in ShoppingCart.java I've used method signature:
public class ShoppingCart {
public void addItem(Product item) {
System.out.printf("Adding %s to the cart.\n", item.getName());
}
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
Not sure what I'm missing. Can anybody help me see what it is?
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item) {
System.out.printf("Adding %s to the cart.\n", item.getName());
}
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers

Ken Alger
Treehouse TeacherJason;
As Mr. Dennis mentioned, you are close.
One of the things you can do in Java is have multiple functions with the same name that take different parameters. As long as they are defined properly Java will figure out which one you are trying to use. Let's say we are building a house and we need functions for adding doors, for example:
public void addDoor(int number) {
numberOfDoors = numberOfDoors + number;
}
and
public void addDoor(int number, String location) {
numberOfDoors = numberOfDoors + number;
// set location as either interior or exterior
if (location.equals("interior")) {
interiorDoors ++;
}
else if (location.equals("exterior")) {
exteriorDoors ++;
} else {
system.out.println("Um... most doors are either interior or exterior doors.
Where should we put this one?");
}
}
Those both work. If you pass addDoor()
only an integer, it simply increases the number of doors overall without taking into consideration the location. If you pass addDoor()
a number and a location Java looks at both. Now, to simplify our code a bit, since addDoor(int number)
is already defined and addDoor(int number, String location)
uses the same code for part of it's functionality, wouldn't it be great if we could do...
public void addDoor(int number, String location) {
addDoor(number);
// set location as either interior or exterior ... code left out for brevity
You know what? We can! Now if we want to change what happens to the number whenever we add a door (increase the number of door knobs, hinges and door stops for example), we only have to do it in one spot. Both functions are covered.
Back to our challenge, addItem()
is a similar concept.
Hope it helps.
Ken

Craig Dennis
Treehouse TeacherThe addItem method should call the original addItem method that it overloads.
You are so close! Great job!

Jess Sanders
12,086 PointsAll you need to do is change
"Adding %s to the cart.\n"
to
"Adding 1 of %s to the cart.\n"

Craig Dennis
Treehouse TeacherYou should call the addItem
method and pass it a 1. Hackers!

Jess Sanders
12,086 PointsOK, I understand better now. The way some of us are going about solving this challenge creates code duplication, and that's not good. :(
Jason Laux
3,128 PointsJason Laux
3,128 PointsThanks to both Craig and Ken. Great example above! I should have picked that up from the video exercise. I guess that's why we do the the challenges at the end. I understand that the signature of the two is different. But it feels like cheating, sort of like recursion or something. Really enjoying the class, by the way.