Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial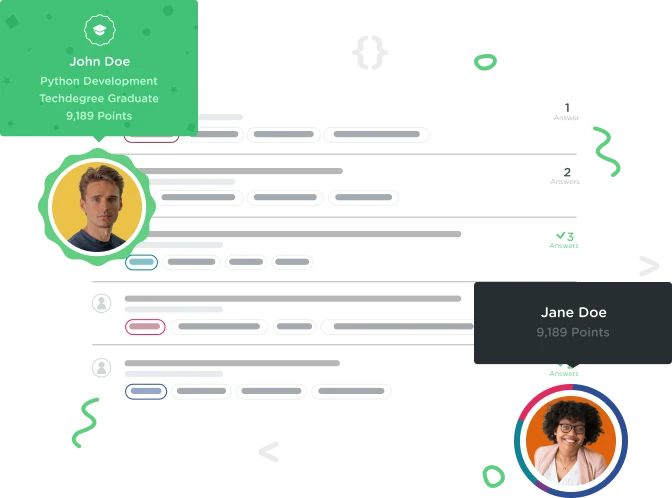
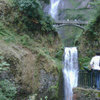
Don Shipley
19,488 PointsJava
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
Where it covered adding arrays to a function?
Sorry tired of looking up information on the net to understand and write the answer. Codes form the video are not covered to my understanding in the video subject. Maybe covered in previous videos such as the array video where it shows how to place a function inside an array, but not covered placing an array inside a function... Is the arguments inside the function become an array?
9 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsHello, Don. First of all, it's very important to understand that JavaScript is not Java. Even though the name might suggest otherwise, apart from some superficial similarities, JavaScript is a completely different language. The name itself is an unfortunate marketing ploy. I'm only pointing this out because of your discussion title.
Now, as for your question, a function in JavaScript can take pretty much anything as a parameter. As you'd pass a string or a number, you can pass an array, object, even another function as a parameter:
var myString = "some string";
var myNumber = 2;
var myArray = [1, 2, 3];
someFunction(myString); // passing a string to the function
someFunction(myNumber); // passing a number to the function
someFunction(myArray); // passing an array to the function
Your task here is to create a function which will accept any parameter, but it will do different things depending on the type of the parameter. If you pass a string, number or undefined
, it will return 0
. If you pass an array, it will return the length of that array (you achieve this by returning the array's length property).
This is what your function must do:
- accept a parameter
- check if it's an array
- if it is an array, return the length of the parameter
- if it's not an array, return
0
The first step is easy, declare the function and let it take a parameter. Let's call it param
:
function arrayCounter(param) {
}
As it is, this function does nothing. Let's change that. To make it return a value, you can use the return
statement:
function arrayCounter(param) {
return 0;
}
This function will now return 0
regardless of what type of the parameter is. Now, let's just check if it's an array and return its length if it is.
function arrayCounter(param) {
if (param instanceof Array) {
return param.length;
}
return 0;
}
We can use the instanceof
operator to check if the parameter we've passed to the function is an array. If it is, we return the length.
There's no need to write an else
statement here as the return
statement ends the execution of the function. If our check doesn't pass, the second return
statement is triggered, the one that returns 0
.
There is also a slightly more verbose solution to this problem (one that is more common on the forum and shown in the video) that goes like this:
function arrayCounter(param) {
if (typeof param !== "string" && typeof param !== "number" && typeof param !== "undefined") {
return param.length;
}
return 0;
}
This one uses the typeof
operator to explicitly check for types that we want to return 0
for.
I hope this helps.
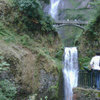
Don Shipley
19,488 PointsSorry I know there is a difference in the lang. Ok Java script inside the tracks I am on. My main point is the questions should be more understandable and only in the past video covered. Using notepad++ this code as well as others work. I do not understand what the instructor wants he only covered strings in this video. I have written the code the exact way from his video change the function name to match and it still fails. With the code below created a var numbers
var numbers = [];
function arrayCounter(numbers) {
if(typeof numbers === 'undefined') {
numbers = 0;
}
return numbers.length;
}
console.log(numbers.length);
His code from what he has taught below.
// Functions
// Arguments
/* function sayHello (name, greeting) {
console.log(greeting + " World " + name);
}
sayHello("Don", "Greetings");
sayHello("Don");
*/
/* function sayHello (name, greeting) {
if (typeof greeting === 'undefined') {
greeting = "Hello";
}
console.log(greeting + " World " + name);
}
sayHello("Don", "Greetings");
sayHello("Don");
*/
// Return Values
/* function sayHello (name, greeting) {
if(typeof name === 'undefined'){
return 0;
}
if (typeof greeting === 'undefined') {
greeting = "Hello";
}
console.log(greeting + " World " + name);
// return "Done";
// return; // will return an undefined
return name.length;
console.log('End of function');
}
// sayHello("Don", "Greetings");
// sayHello("Don");
console.log(sayHello("Robert", "Greetings"));
console.log(sayHello("Don"));
console.log(sayHello());
*/
/*
var = my_array["don"]
function arrayCounter(name) {
if(typeof name === 'undefined'){
name = 0;
}
if (typeof greeting === 'undefined') {
greeting = "Hello";
}
return arrayCounter.length;
console.log(name, greeting)();
}
console.log(arrayCounter());

Dino Paškvan
Courses Plus Student 44,108 PointsYou get the length of an array the same way you get the length of a string. It's a property you can access with the dot notation (someArray.length
). Basically, the code challenge wants you to take one step further and apply the same steps to a different data type.
I have provided you with a detailed explanation of the solution above. If you go through it, you'll see that it behaves in the same way, only it checks for different data types.
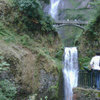
Don Shipley
19,488 PointsI you are speaking of the last code I should have deleted it from the reply. This is one I was just trying using notepad++ and not that will not work. After trying several different ways of writing the code nothing works passing the test question including trying your code you wrote earlier.
Using notepad++ this code will show me the length being 0 yet still fails on the test question.
function arrayCounter(name) {
if(typeof name === 'undefined'){
return 0;
}
return name.length;
console.log(name());
}
console.log(arrayCounter());
It would be nice if you could skip the one question to continue with the test... It would also be nice if the test question dealt with the immediate previous video. Trying others now

Samara Soucy
6,733 PointsDon,
To be fair, I had some trouble with this challenge as well, but it was actually covered in the video. Your last code was:
var numbers = [];
function arrayCounter(numbers) {
if(typeof numbers === 'undefined') {
numbers = 0;
}
return numbers.length;
}
console.log(numbers.length);
The problem is that instead of returning 0 if numbers is undefined as per the instructions, you are setting numbers to 0 and then returning its length, which is not what you want. Instead try:
var numbers = [];
function arrayCounter(numbers) {
if(typeof numbers === 'undefined') {
return 0;
}
return numbers.length;
}
console.log(numbers.length);
If you expand that out to also check for numbers and strings before returning the length of the array you should pass no problem.
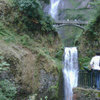
Don Shipley
19,488 PointsSorry no the above answer does not pass the test question
no code I have tried will pass the test question. Including the instructors code form the video.
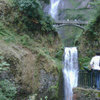
Don Shipley
19,488 Pointsusing the below code will show me a 0. Have not gotten to document.write in the videos yet so can replace the document.write with console.log and will show the same below the code is the question on the test...
function arrayCounter (name) {
if (typeof name === 'undefined'){
return 0;
}
return name;
return name.length;
}
document.write (arrayCounter());
TEST QUESTION, Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.

Dino Paškvan
Courses Plus Student 44,108 PointsI don't know if you've solved this yet or not, but my first post actually contains code you can just paste into the challenge and pass it.
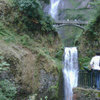
Don Shipley
19,488 PointsThank you Dino for your input.. Your code is a little advanced for the stage I am at. I believe Jim Hoskins has spoken of !== to in another video but not the one I am watching at this time. I wrote the code to my understanding of the video where it is using strings. Using notepadd++, Chrome and the console.log. My code does return a 0 value. I may be miss reading the question or I am just to ignorant to understand. I will copy your code and try it in the test question.
Thank you for all your help.. Don
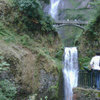
Don Shipley
19,488 PointsHi Dino, Again thank you for all your help. Your response helped me a great deal. The past couple days I have been re watching the videos. With you response I came to the conclusion that this is the answer he is looking for on the test question, since on this serious of videos he has not covered the not statement.
function arrayCounter(name) {
if (typeof name == 'undefined'){
return 0;
}
if (typeof name == "string"){
return 0;
}
if (typeof name == "number"){
return 0;
}
return name.length;
}
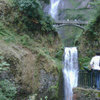
Don Shipley
19,488 PointsHi Dino, Again thank you for all your help. Your response helped me a great deal. The past couple days I have been re watching the videos. With you response I came to the conclusion that this is the answer he is looking for on the test question, since on this serious of videos he has not covered the not statement.
function arrayCounter(name) {
if (typeof name == 'undefined'){
return 0;
}
if (typeof name == "string"){
return 0;
}
if (typeof name == "number"){
return 0;
}
return name.length;
}