Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial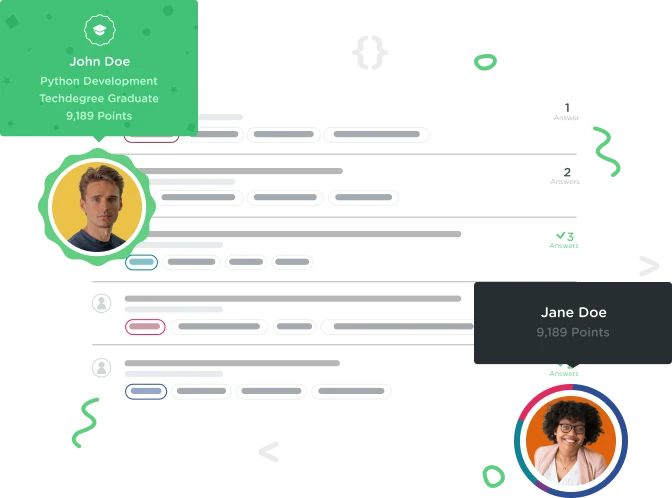

Damjan Vlaic
19,244 Points---JAVA---
i don't understand how to do this; i would appreciate help :)
Now make sure that if a com.example.BlogPost is passed in for obj that you then cast it to a BlogPost. Return the results of the getTitle method on the newly type-casted BlogPost instance. I've included BlogPost.java for your reference only
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (obj instanceof String) {
result = (String) obj;
}
return result;
}
}
2 Answers
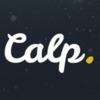
calp
10,317 PointsYou just need to do what you've already done for the String but with the BlogPost object instead,
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if (obj instanceof String) {
result = (String) obj;
} else if(obj instanceof BlogPost) {
BlogPost bp = (BlogPost) obj;
result = bp.getTitle();
}
return result;
}
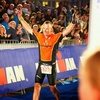
Steve Hunter
57,712 PointsCorrect but if you look at the specification, the obj
object can only exist in two states; a String
or a BlogPost
. That said, there's no need to directly test the second condition; if the first condition fails, then obj
isn't a String
so it must be a BlogPost
.
You can just do the cast in the else
clause. You can use the result
variable to hold each respective result and return it after the if
conditional has run, or you can return either option directly within each condition. Having two return statements is sometimes frowned upon, but where they are mutually exclusive, i.e. both can never run, it's less of an offence.
if(obj instanceof String){
return (String) obj;
} else {
return ((BlogPost) obj).getTitle();
}
Alternatively, do as above and set result
to the cast object and return result
at the end of the method.
Steve.

Damjan Vlaic
19,244 Pointstnx for helping steve :)
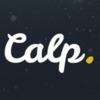
calp
10,317 PointsThe result variable is provided by the challenge, two returns do look better though. My only thing would be the fact that it might not be a BlogPost. I know the challenge states it will be but I would get a slap on the wrist for not checking for that.
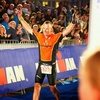
Steve Hunter
57,712 PointsHi Cal,
Yes, result
is part of the question - I found it redundant but others prefer the single return
statement. I think the single return
is probably better practice but, like you, I just prefer the look of the other solution. If the code was any longer, I think it would become confused so setting result
would be the better option clarity-wise.
I take the challenges literally - but there's nothing wrong with making the additional check; I was just pointing out the potential unnecessary code - again, if the solution was any more complicated, the explicit check would definitely be a clearer solution. I would get a slap on the wrist for "not adhering to the defined requirements" and for running additional overhead that's not needed - but that probably says more about my customers than anything else! I can hear them now!
All good discussion - I think exploring different solutions to the same problems helps us all think more broadly. I think the solution I suggested works fine for this challenge but it probably lacks clarity that the longer solution provides. And the additional overhead is. obviously, negligible and irrelevant in this scenario. The double check provides greater clarity and, as you say, safeguards this chunk of code should other things change. The shorter solution is very specific and could break should anything else alter.
So, in all, your solution is probably better all round; my offering is good for discussion purposes only, perhaps. It's probably bad practice on two fronts!
Steve.
Damjan Vlaic
19,244 PointsDamjan Vlaic
19,244 Pointstnx for helping Cal, you helped a lot ;)