Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial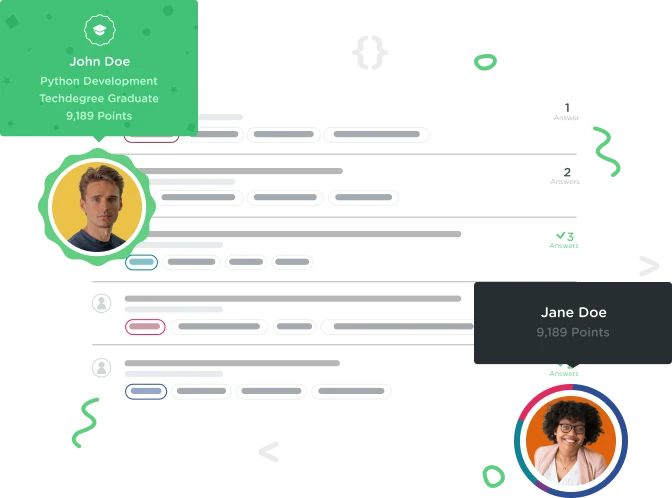

Jodie Wong
3,522 PointsJava Android Array
Hi
I'm doing the extra credit bit of this module. Have the app reverse the order of numbers on screen when the button is pressed.
I've got the code working it's great and all but there's one bit I'm a bit unsure of:
public class NumberSwapActivity extends Activity {
public void arraySwap( int array[] ){
int temp;
temp = array[0];
array[0] = array[3];
array[3] = temp;
temp = array[1];
array[1] = array[2];
array[2] = temp;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_number_swap);
//
final TextView firstNumber = (TextView)findViewById(R.id.firstNum);
final TextView secondNumber = (TextView)findViewById(R.id.secondNum);
final TextView thirdNumber = (TextView)findViewById(R.id.thirdNum);
final TextView fourthNumber = (TextView) findViewById(R.id.fourthNum);
Button swapNumButton = (Button)findViewById(R.id.swapNumButton);
final int numArray[] = {1,2,3,4};
View.OnClickListener Listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
firstNumber.setText( numArray[ 0 ] + "" );
secondNumber.setText( numArray[ 1 ] + "" );
thirdNumber.setText( numArray[ 2 ] + "" );
fourthNumber.setText( numArray[ 3 ] + "" );
arraySwap(numArray);
}
};
swapNumButton.setOnClickListener(Listener);
}
So as I understand from Ben's previous vids, I do need the numArray as final since I'm referencing it from the OnClickListener. Final also means that the array is constant and yet I can still use arraySwap to do what I want.
So how is Java letting arraySwap diddle around with the numArray?
I have 2 theories:
Since I have tried defining the arraySwap function within the onCreate block with no success, I am lead to the conclusion that arraySwap is able to work since it's not in the same code block/scope.
Maybe arraySwap doesn't actually do anything to numArray and just manipulates the parameter it's fed rather than the original array itself.
So if anyone can help clarify things, that would be awesome.
Thanks
1 Answer
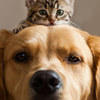
Devin Scheu
66,191 PointsSo the way you did this was right. Your first assumption is right, because of scope that function cannot be called on the OnCreate method. And your second is also correct, if you wanted it to manipulate the array its self you would have to store the arraySwap back into the variable. The function simple uses the parameters it is passed. I Hope this helps :)!