Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial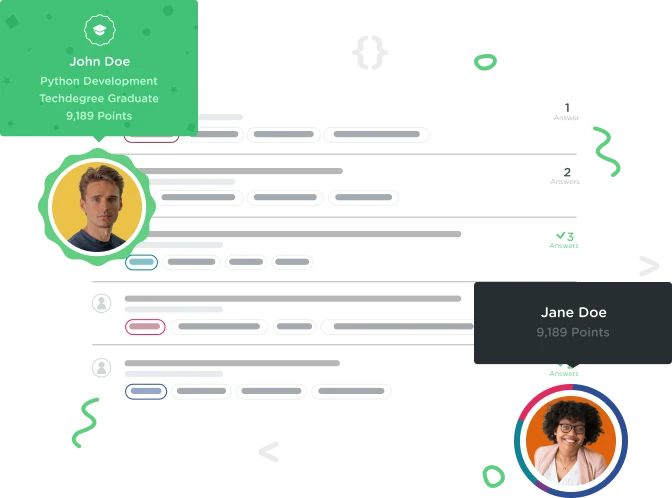

Steven Mathews
2,200 PointsJava Basics - Coding the Prototype
I can tell that there (surely) would be a much better way of doing this.. but with my limited Java skills (and a bit of looking around the web), I came up with this to use "an" if the adjective is a vowel:
char firstLetter = adjective.charAt(0);
if (firstLetter == 'a' || firstLetter == 'e' || firstLetter == 'i' || firstLetter == 'o' || firstLetter == 'u') {
console.printf("Your TreeStory:\n-----------\n");
console.printf("%s is an %s %s. ", name, adjective, noun);
console.printf("\n%s is always %s %s.\n", name, adverb, verb);
}
else {
console.printf("Your TreeStory:\n-----------\n");
console.printf("%s is a %s %s. ", name, adjective, noun);
console.printf("\n%s is always %s %s.\n", name, adverb, verb);
}
2 Answers

Craig Dennis
Treehouse TeacherNice job! There are definitely better ways to do that, but excellent job sniffing around! One thing for sure that you could clean up here, which Steven mentions is the DRY (Don't Repeat Yourself) problem of the multiple lines.
Try making the if
and else
code blocks print out just the code that is different.
Again, awesome job! Keep at it! You'll pick up more tricks shortly that will make this even more possible!

Steven Mathews
2,200 PointsFixed it all up (you might notice I commented on my own work above ^ 8P )..
I'm going to put in a question about sex, and use he/she accordingly.
But it's getting late ; P this'll do for now.
Also chucked the Extra Credit stuff in there:
//get the age, and check that it's 13 or over. Else exit
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
console.printf("Sorry, you must be at least 13 to use this program.\n");
System.exit(0);
}
//get the name
String name = console.readLine("Enter a name: ");
//get an adjective, check if it starts with a vowel, if so use "an", else use "a"
String adjective = console.readLine("Enter an adjective: ");
char adj = adjective.charAt(0);
String adjectiveFirstLetter;
String vowels = "aeiou";
String article;
if (vowels.contains (String.valueOf(adj))) {
article = ("an");
}
else {
article = ("a");
}
//get a noun, check if it's in our list of naughty words. if so, say so and ask for another
String noun;
String invalidWords = ("dorknerdjerkbuttface");
boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = invalidWords.contains(noun.toLowerCase());
if (isInvalidWord) {
console.printf("That language is not allowed. \n Try again. \n\n");
}
} while (isInvalidWord);
//get an adverb and a verb
String adverb = console.readLine("Enter an adverb (usually ends with -ly): ");
String verb = console.readLine("Enter a verb (usually ends with -ing): ");
//print story using all input items from above
console.printf("Your TreeStory:\n-----------\n");
console.printf("%s is %s %s %s. ", name, article, adjective, noun);
console.printf("\n%s is always %s %s.\n", name, adverb, verb);

May Loh
5,213 PointsCould someone help shed some light on this? I'm very new to programming. I'm trying to understand the code but I'm having some difficulty.
What is "adj in "char adj = adjective.charAt(0);"? I didn't see it being declared as a string at the top but I can't find it online as a java syntax. And what does this line do?
Also, I get that "vowels.contains" is looking for "aeiou" but what does "String.valueOf(adj)" do?
I'm trying to work out my own code too, and I keep getting this error: variable isInvalidWord is already defined in method main(String[]). I also keep getting this error: incompatible types: String cannot be converted to boolean.
Advice would be gladly appreciated.

Steven Mathews
2,200 PointsInstead of String (which holds a 'string' of letters), char holds a specific character.
http://docs.oracle.com/javase/7/docs/api/java/lang/Character.html
In this case, I am declaring a char, naming it "adj", and setting it to be the first (0) letter of the string "adjective" (which is set to be whatever the user types in to the prompt "Enter an adjective: ")
^ that should answer your next question too (String.valueOf changes it from a char to a String)
for help with your code, would need to have a look at it (check out "Tips for asking questions", over there --> for help on including your code in the forums)

May Loh
5,213 PointsHi Steven,
I think I get what you mean. I also tried to insert the vowels as a list but it didn't work.
Here's my code:
```import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here! */
//--Name-- is a --adjective-- --noun--. They are always --adverb-- --verb--.
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code
console.printf("Sorry, you must be at least 13 to use this program.\n");
System.exit(0);
}
//Start the sequence, get the name
String name = console.readLine("Enter a name: ");
//Get the adjective
String adjective = console.readLine("Enter an adjective: ");
//Print the article word if the adjective starts with aeiou
String article;
String vowels = "aeiou";
if (adjective.charAt(0) == "a" || "e" || "i" || "o" || "u") {
article = ("an");
}
else {
article = ("a");
}
//Getting the noun.
String noun;
//This is a variable and checks if the variable contains any censored words
boolean isInvalidWord;
//These is the list of censored words
String badWord = "nerd jerk dork idiot";
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = badWord.contains(noun.toLowerCase());
if (isInvalidWord) {
console.printf("That language is not allowed. Try again. \n\n");
}
} while (isInvalidWord);
String adverb = console.readLine("Enter an adverb: "); String verb = console.readLine("Enter a verb ending with -ing: ");
console.printf("Your TreeStory\n-------------------\n");
console.printf("%s is %s %s %s.\n", name, article, adjective, noun);
console.printf("He is always %s %s.\n", adverb, verb);
//get the age, and check that it's 13 or over. Else exit
/* String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
console.printf("Sorry, you must be at least 13 to use this program.\n");
System.exit(0);
}
//get the name String name = console.readLine("Enter a name: "); //get an adjective, check if it starts with a vowel, if so use "an", else use "a" String adjective = console.readLine("Enter an adjective: "); char adj = adjective.charAt(0); String adjectiveFirstLetter; String vowels = "aeiou"; String article; if (vowels.contains (String.valueOf(adj))) { article = ("an"); } else { article = ("a"); } //get a noun, check if it's in our list of naughty words. if so, say so and ask for another String noun; String invalidWords = ("dork nerd jerk buttface"); boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = invalidWords.contains(noun.toLowerCase());
if (isInvalidWord) {
console.printf("That language is not allowed. \n Try again. \n\n");
}
} while (isInvalidWord);
//get an adverb and a verb
String adverb = console.readLine("Enter an adverb (usually ends with -ly): ");
String verb = console.readLine("Enter a verb (usually ends with -ing): ");
//print story using all input items from above
console.printf("Your TreeStory:\n-----------\n");
console.printf("%s is %s %s %s. ", name, article, adjective, noun);
console.printf("\n%s is always %s %s.\n", name, adverb, verb); */
}
}
Steven Mathews
2,200 PointsSteven Mathews
2,200 Pointsi gather it would be something to do with making an array of vowel chars and using .compare(), and maybe for just the letter n concatenating in a variable (not using DRY there)