Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial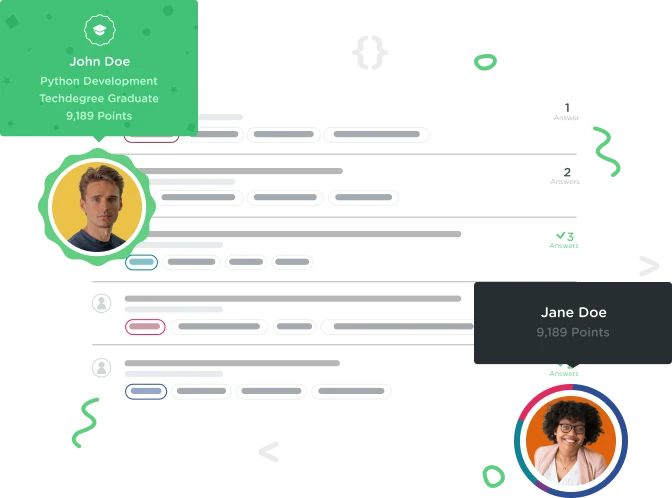

Shane McC
3,005 PointsJava - Cannot be resolved to a variable?
Hi Everyone,
I'm getting this weird error and eclipse is telling me my finalAmount variable can't be resolved. From looking at it I know it's outside of the scoop of the for statement and everytime I create a local variable and assign a value to it (it's zero) my program gets messed up.
My question is, how would I declare the finalAmount variable locally?
Thanks
import java.util.Scanner;
public class CompoundInterest {
public static void main(String[] args){
double rate;
double amount;
double year;
System.out.println("This program, with user input, computes interest.\n" +
"It allows for multiple computations.\n" +
"User will input initial cost, interest rate and number of years.");
Scanner input = new Scanner(System.in);
System.out.println("What is the inital cost?");
amount = input.nextDouble();
System.out.println("What is the interest rate?");
rate = input.nextDouble();
rate = rate/100;
System.out.println("How many years?");
year = input.nextDouble();
for(int x = 1; x < year; x++){
double finalAmount = amount * Math.pow(1.0 + rate, year);
// the below works but the problem is, it prints the statement out many times. I don't want that.
/* System.out.println("For " + year + " years an initial " + amount +
" cost compounded at a rate of " + rate + " will grow to " + finalAmount); */
}
System.out.println("For " + year + " years an initial " + amount +
" cost compounded at a rate of " + rate + " will grow to " + finalAmount);
}
}
1 Answer

omars
9,156 PointsShane,
Q: "eclipse is telling me my finalAmount variable can't be resolved" A: This is because you are declaring 'finalAmount' within the for loop. Once your for loop exits, 'finalAmount' goes out of scope. Meaning, Java has no clue it ever existed.
Q: "My question is, how would I declare the finalAmount variable locally?" A: From what I know, you cannot declare a variable within a loop of any kind if you want to retain the previous value. When you declare a variable within a loop this is what happens:
- Your loop begins with an initial value of 0. (This is before the calculation takes place, double finalAmount;)
- A value is calculated and assigned to finalAmount.
- Your loop ends.
- If you loop condition is still valid (x < year), repeat from step one (finalAmount is redeclared and initialized).
Someone please correct me if I said anything wrong about the above steps.
Here is my suggested change to your code, I hope this helps.
import java.util.Scanner;
public class CompoundInterest {
public static void main(String[] args){
double rate;
double amount;
double year;
System.out.println("This program, with user input, computes interest.\n" +
"It allows for multiple computations.\n" +
"User will input initial cost, interest rate and number of years.");
Scanner input = new Scanner(System.in);
System.out.println("What is the inital cost?");
amount = input.nextDouble();
System.out.println("What is the interest rate?");
rate = input.nextDouble();
rate = rate/100;
System.out.println("How many years?");
year = input.nextDouble();
/* Calculate the interest over a number of 'years' and
assign the value to 'finalAMount'
*/
double finalAmount = 0; // Perfectly legal to do this since finalAmount isn't used prior to this.
for(int x = 1; x < year; x++){
finalAmount = amount * Math.pow(1.0 + rate, year);
}
System.out.println("For " + year + " years an initial " + amount +
" cost compounded at a rate of " + rate + " will grow to " + finalAmount);
}
}
Shane McC
3,005 PointsShane McC
3,005 PointsOmar,
Thanks. That worked great but I'm trying to figure out, what's the difference between your syntax and mine? I've been looking at this for a while and can't quite seem to place it. Thanks again.
Shane McC
3,005 PointsShane McC
3,005 PointsOmar,
Thanks. That worked great but I'm trying to figure out, what's the difference between your syntax and mine? I've been looking at this for a while and can't quite seem to place it. If I place the finalAmount variable above my syntax it's giving an error message of duplicate variable but in your syntax it's not. How come? Thanks again.