Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial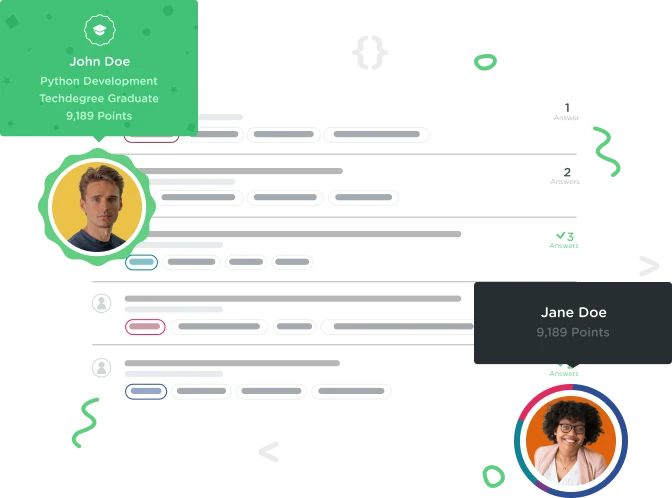

Daniel Silva
5,353 PointsJava can't find my file
I wrote this program that reads in lines from a csv file. When I run the code, I get an error message below. The CSV file is in the src folder so I don't know what the issue is.
package books;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class BookApp {
public static void main(String[] args){
List<Book> b = Book.getList("src/books.csv");
System.out.println();
System.out.println("Sorted List:");
Collections.sort(b);
for(Book book: b){
System.out.println(book.toString());
}
Comparator c = Collections.reverseOrder();
Collections.sort(b, c);
System.out.println();
System.out.println("Reverse Order:");
for(Book book: b){
System.out.println(book.toString());
}
}
}
package books;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class Book implements Comparable<Book> {
private String title;
private String author;
private int year;
public Book(String title, String author, int year){
this.title=title;
this.author=author;
this.year=year;
}
public String getTitle(){
return title;
}
public String getAuthor(){
return author;
}
public int getYear(){
return year;
}
@Override
public String toString(){
return title+" by "+author+'('+year+')';
}
public static List<Book> getList(String f){
List<Book> books = new ArrayList<>();
int bookNo = 0;
File file = new File(f);
Scanner input;
try {
input = new Scanner(file);
while(input.hasNext()){
String[] parts = input.nextLine().split(",");
if(parts.length==3){
Book b = new Book(parts[0],parts[1],Integer.parseInt(parts[2]));
books.add(b);
bookNo++;
}else{
System.out.println("Problem reading: "+Arrays.toString(parts).replace("[","").replace("]", ""));
}
}
input.close();
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("Number of books: "+bookNo);
return books;
}
@Override
public int compareTo(Book o) {
return this.getTitle().compareTo(o.getTitle());
}
}```
2 Answers

Ernest Rodriguez
17,643 PointsI ran your code as is and it compiled without errors. Right-click the .csv file in the IntelliJ project Window and select "Copy Path". This will give you the absolute path of the file which you can then paste as the argument for your function
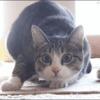
Jacob Armstrong
4,352 PointsSince the .csv file is in the classpath, you do not need to include /src in your file name. Just use "/books.csv".

Daniel Silva
5,353 PointsJacob Armstrong I've tried that too. It's driving me crazy....
Fernando Boza
25,384 PointsFernando Boza
25,384 PointsTHANK YOU!