Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial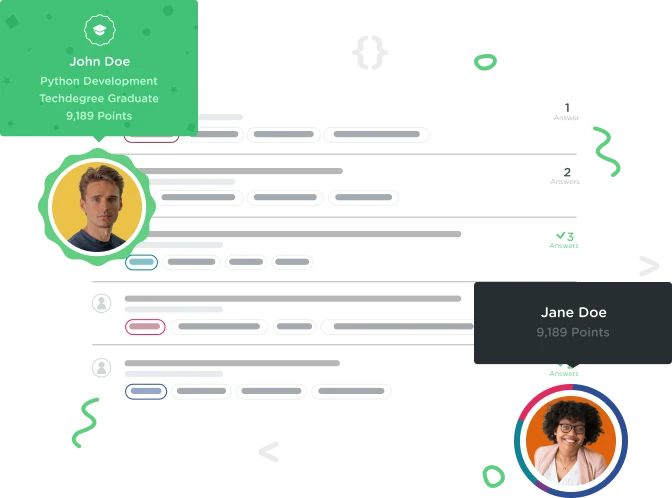

Nicholas Anstis
2,095 PointsJava Challange getting this error "cannot assign a value to final variable"
Here's my code and for some reason the "final" keyword mess up something.
public class GoKart {
public static final int MAX = 8;
private String mColor;
public final int mGoKart;
public GoKart(String color) {
mColor = color;
}
public void load() {
mGoKart = MAX;
}
public String getColor() {
return mColor;
}
}
5 Answers
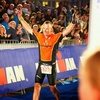
Steve Hunter
57,712 PointsHi Nicholas,
Let's walk through the challenge. First, Let's add a constant field to the class that stores the maximum number of energy bars. You've done this right with your line:
public static final int MAX = 8;
Next, Now let's add a private uninitialized field to store the current number of energy bars. Name it mBarsCount
. Initialize it to zero in the constructor. So, we need to create a new member variable with a given name. It holds an integer and is private. We set it to zero in the constructor. So, at the top of the class:
public class GoKart {
private String mColor;
public static final int MAX = 8;
private int mBarsCount; // added here
Then, in the constructor:
public GoKart(String color) {
mColor = color;
mBarsCount = 0; // added this line
}
The, lastly, let's add a method named charge. It should be public and return nothing. When called it should set mBarsCount
to the value of the constant you declared in Task 1. So, we need a new method that sets mBarsCount
to MAX
. It is public, returns nothings, takes no parameters and is called charge
:
// whole new method
public void charge(){
mBarsCount = MAX;
}
And that's it!
I hope that helps,
Steve.
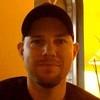
Jeremy Hill
29,567 PointsThis line you have might be what is causing your problem:
public final int mGoKart;
There shouldn't be a final there, or an int:
public GoKart(String color){
}

Julian Garcia
18,380 Pointsfinal keyword is used for constants, where they are defined they need to assign a value and it cannot be changed later.
So try changing to this:
private int mBarsCount; //They ask to be a private variable
instead
public final int mGoKart; // what's this for, Julian?
the function you name load , they ask to name it charge, so just change the name.
They also ask to initialize the variable mBarsCount to zero in constructor, GoKart method and add
public GoKart(String color) {
mColor = color;
//Here assign zero to mBarsCount
}
Hope this help.
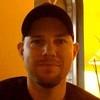
Jeremy Hill
29,567 PointsYour final declaration should look like this:
public static final int MAX_BARS = 8;

Nicholas Anstis
2,095 PointsOh I get it. Thanks a lot

Nicholas Anstis
2,095 PointsActuall it doesn't work. Changing it doesn't do anything.
And the error is in the public final int mGoKart;
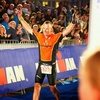
Steve Hunter
57,712 PointsYou also need to remove the line:
public final int mGoKart;
That's the final
that is causing the problem as you are later trying to assign to that variable in your load
method where you set mGoKart = MAX
. You can't assign to a final
variable except on the same line you declare it.
So, public final int mGoKart = MAX;
is fine (but not the solution), but assigning to mGoKart
later isn't allowed.
I've written a walk-through of the challenge step-by-step. I hope that helps.

Nicholas Anstis
2,095 PointsThanks you all of you. I got this right :]