Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial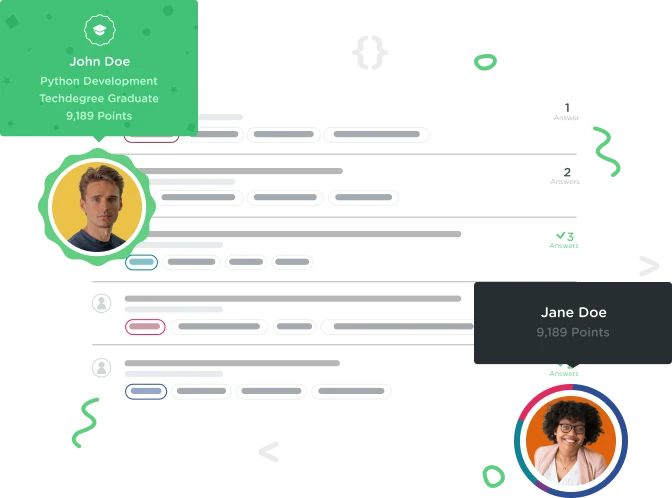

Arthur Podkowiak
3,633 PointsJava Challenge: Defaulting Parameters code not working
Why is my written code not working? I have clearly changed the signature of the addItem method, but changed it to only call the item name, and not the second argument, quantity of the item. Does this not mean that when someone uses the method and inputs only an item name but no quantity the code should run the method with one argument and not the method with 2 arguments? I'm so confused why this isn't working.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product item) {
System.out.printf("Adding 1 %s to the cart.\n", item.getName());
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers
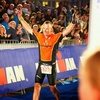
Steve Hunter
57,712 PointsYou want to have a 1 in there instead of quantity
- you've not got anything in there, so:
public void addItem(Product item) {
System.out.printf("Adding %d of %s to the cart.%n", 1, item.getName());
/* Other code omitted for clarity */
}
Hope that makes sense!
Steve.

Arthur Podkowiak
3,633 PointsAha! Thank you Steve, if you do look at my comment above though, I passed using a different way. This makes much more sense, I always thought that %s or %d or similar things had to call variables at the end of the printf statements.
Thanks so much!
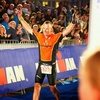
Steve Hunter
57,712 PointsI saw you'd got through it, which is the main aim!
I'm pretty sure I remember doing that challenge and passing it in that way - I may well be wrong! The % interpolators just need to refer to the correct type, so an int or String, so they can be literals or variables of that type.
Steve.

Craig Dennis
Treehouse TeacherI've modified this challenge, you now need to call the method that you are overriding, as I had originally intended.

Liron Tal
4,825 PointsThis should also work:
System.out.printf("Adding 1 %s to the cart.\n", item.getName());
But doesn't :(
Liron.
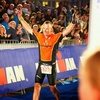
Steve Hunter
57,712 PointsNo, this challenge was changed, as Craig said above.
You need to call the overriden method from inside the new one.
There's a full explanation in this thread.
Let me know how you get on.
Steve.
Arthur Podkowiak
3,633 PointsArthur Podkowiak
3,633 PointsSo I just passed this challenge. The reason why my code wasn't working was because I changed the System.out message from:
"Adding 1 %s to the cart."
to
"Adding 1 of %s to the cart."
I think this needs to be called to attention, as it grammatically makes more sense to say "I'm buying 1 Yoda dispenser" rather than "I'm buying 1 OF Yoda dispenser". I am happy I found my error, but I thing this bug and bugs just like it should be called to attention, otherwise users spend hours trying to fix their code when it doesn't actually need fixing.