Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial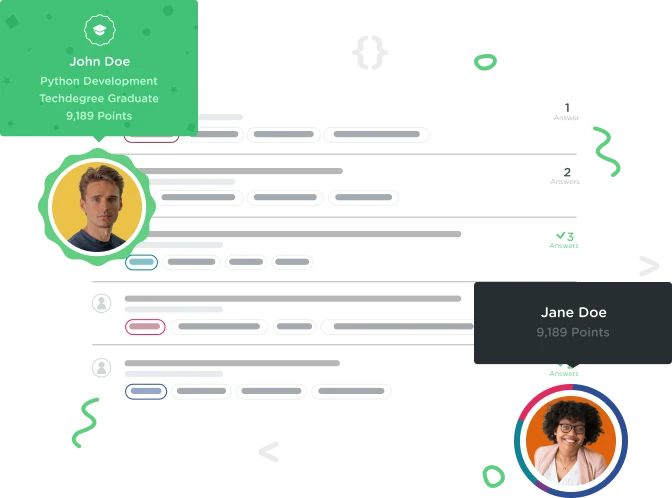
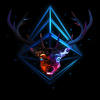
Anthony Ramnarain
8,951 PointsJava Closure Code Challenge
public static Function<Integer, String> getArgentinaPriceConverter() { Locale argLocale = Locale.forLanguageTag("es-AR"); // This fluctuates and is hardcoded temporarily BigDecimal argPesoToUsdRate = new BigDecimal("15.48");
Function<Integer, BigDecimal> usdToArgentinePesoConverter =
usd -> argPesoToUsdRate.multiply(new BigDecimal(usd));
Function<BigDecimal, String> argentineCurrencyFormatter = price -> {
Currency currentCurrency = Currency.getInstance(argLocale);
NumberFormat currencyFormatter =
NumberFormat.getCurrencyInstance(argLocale);
return String.format("%s (%s)",
currencyFormatter.format(price),
currentCurrency.getDisplayName()
);
};
return usdToArgentinePesoConverter.andThen(argentineCurrencyFormatter);
}
public static Function<Integer, String> createPriceConverter(Locale locale, BigDecimal usdRate) {
// TODO: Examine the hardcoded `getArgentinaPriceConverter` method
// TODO: Using the arguments passed into this method return a function that will work for any locale and rate
return usdPrice -> {
Currency currentCurrency = Currency.getInstance(locale);
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
return String.format("%s (%s)", currencyFormatter.format(usdRate.multiply(new BigDecimal(usdPrice))), currentCurrency.getDisplayName());
};
}
public static Function<Integer, String> createPriceConverter(Locale locale, BigDecimal usdRate) {
// TODO: Examine the hardcoded `getArgentinaPriceConverter` method
// TODO: Using the arguments passed into this method return a function that will work for any locale and rate
return usdPrice -> {
Currency currentCurrency = Currency.getInstance(locale);
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
return String.format("%s (%s)", currencyFormatter.format(usdRate.multiply(new BigDecimal(usdPrice))), currentCurrency.getDisplayName());
};
}

Unsubscribed User
1,321 PointsI formatted your code so its more readable.
public static Function<Integer, String> getArgentinaPriceConverter() {
Locale argLocale = Locale.forLanguageTag("es-AR"); // This fluctuates and is hardcoded temporarily BigDecimal argPesoToUsdRate = new BigDecimal("15.48");
Function<Integer, BigDecimal> usdToArgentinePesoConverter = usd -> argPesoToUsdRate.multiply(new BigDecimal(usd));
Function<BigDecimal, String> argentineCurrencyFormatter = price -> {
Currency currentCurrency = Currency.getInstance(argLocale);
NumberFormat currencyFormatter =
NumberFormat.getCurrencyInstance(argLocale);
return String.format("%s (%s)",
currencyFormatter.format(price),
currentCurrency.getDisplayName()
);
};
return usdToArgentinePesoConverter.andThen(argentineCurrencyFormatter);
}
public static Function<Integer, String> createPriceConverter(Locale locale, BigDecimal usdRate) {
// TODO: Examine the hardcoded `getArgentinaPriceConverter` method
// TODO: Using the arguments passed into this method return a function that will work for any locale and rate
return usdPrice -> {
Currency currentCurrency = Currency.getInstance(locale);
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
return String.format("%s (%s)", currencyFormatter.format(usdRate.multiply(new BigDecimal(usdPrice))), currentCurrency.getDisplayName());
};
}
public static Function<Integer, String> createPriceConverter(Locale locale, BigDecimal usdRate) {
// TODO: Examine the hardcoded `getArgentinaPriceConverter` method
// TODO: Using the arguments passed into this method return a function that will work for any locale and rate
return usdPrice -> {
Currency currentCurrency = Currency.getInstance(locale);
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
return String.format("%s (%s)", currencyFormatter.format(usdRate.multiply(new BigDecimal(usdPrice))), currentCurrency.getDisplayName());
};
}
1 Answer

lukej
34,222 PointsI get the same AssertionError.
You can fix it by adding this line to the getPriceConversionForRecord()
method: .replace("$\u00a0", "$")
.
return String.format("%s is %s (USD) which is %s",
record.getRegionName(),
usFormatter.format(record.getCurrentHomeValueIndex()),
priceConverter.apply(record.getCurrentHomeValueIndex())
).replace("$\u00a0", "$");
Anthony Ramnarain
8,951 PointsAnthony Ramnarain
8,951 PointsThe error I am receiving java.lang.AssertionError: Expected: is "First is $1.00 (USD) which is $15,48 (Argentine Peso)" but: was "First is $1.00 (USD) which is $Â 15,48 (Argentine Peso)"