Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial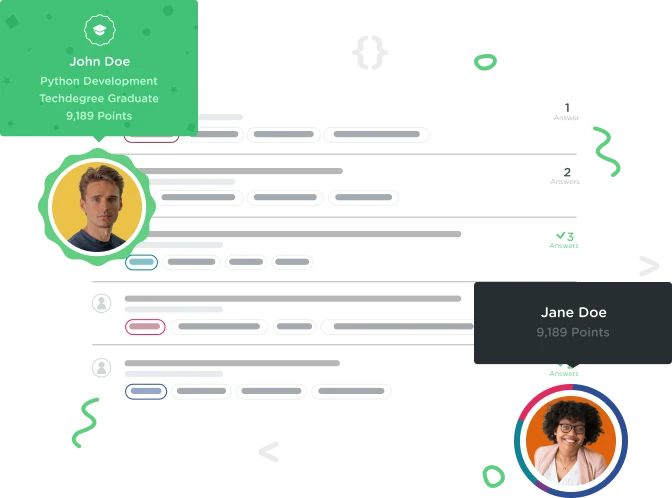
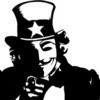
behar
10,799 PointsJava, comparable classes.. Im confused.
Im kind of lost here, i get the idea of implementing features your class can perform, but i dont understand how to implement it. I have the code:
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public int compareTo(Object obj) {
if (equals(obj)) {
return 0;
}
if (obj.compareTo(mCreationDate) == 1) {
// I dont even know what im trying to do...
}
return 1;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
But can someone give me some code and an explanation of how exacly this works, i dont know where to go from here, and i dont really understand what the if (equals(obj)) even means. Help would be much appreciated!
1 Answer
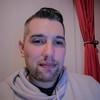
Daniel Hartin
5,311 PointsHi Behar,
the Comparable interface is used to order elements with a Collection like a List. Essentially the computer uses this method to compare items against one another to decide where they should appear within the order. It is expecting a value less than zero for items which should appear further down the list, a value greater than 1 for items which should appear higher in the order and 0 for items which match the order.
Usually you would implement your own logic to determine how you want them ordering and this can vary massively so depends how you want it to work really.
I assume in your example you are tryting to order them by creationDate, the Date class has it's own compareTo method here which you can use
https://www.tutorialspoint.com/java/util/date_compareto.htm
I don't know how it's implemented in the background but how I would approach this is to perhaps take the epoch time of each date which is a long showing how many seconds each is past 01/01/1970 and then subtract one away from the other to get either a value which is negative for an earlier date, positive for a date in the future or 0 if they are the same second exactly.
I hope this helps, but I can be terrible at explaining these things :)
Daniel