Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial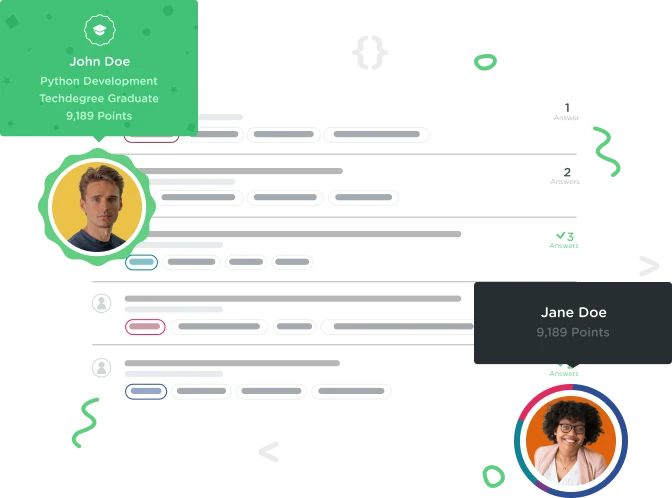

garylacumbre
12,265 PointsJava compile problem
I am running a basic java program in workspaces and it compiles and runs just fine:
import java.util.Scanner;
public class number {
public static void main(String[] args) {
Scanner input = new Scanner( System.in );
int number1;
System.out.print("Enter first integer: "); number1 = input.nextInt();
System.out.printf("You entered number %s \n", number1); } }
But when I try to compile and run it in TextMate for Mac I get this:
Exception in thread "main" java.util.NoSuchElementException at java.base/java.util.Scanner.throwFor(Scanner.java:858) at java.base/java.util.Scanner.next(Scanner.java:1497) at java.base/java.util.Scanner.nextInt(Scanner.java:2161) at java.base/java.util.Scanner.nextInt(Scanner.java:2115) at number.main(number.java:12) Enter first integer:
I am copying the code directly from my Software Engineering lecturer. No idea why it is not compiling. Any help would be much appreciated.
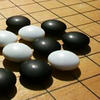
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsNope, System.out.print is correct. It just prints the String and stays on the same line (waiting for user input).
2 Answers
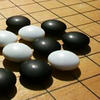
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsYour code compiles and runs on my computer. Check if it really is identical to what you posted, did you maybe delete one character by accident? Or maybe there is some issue with TextMate? I don't have a Mac so I can't test that.

garylacumbre
12,265 PointsThanks for the reply. The code I am compiling is identical so that's not the problem. I am glad to know it works for you as I was worried that my lecturer would not be able to run it. The code runs fine in Workspaces, so it is possibly a TextMate/ Mac issue.
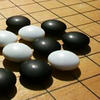
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsSo I searched some more. It seems this code can run into issues because of:
number1 = input.nextInt();
This means your program waits for input and expects the input to be an int. If the input is empty or isn't an int, Bad Things happen, because this is a very simple program that doesn't deal with these cases. The reason why you get an error with empty input and I don't is probably because you're on a Mac and I'm on Windows, they have slightly different ways to deal with input.
There are ways to deal with it. A simple option is:
import java.util.Scanner;
public class Number {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int number1 = 0;
System.out.print("Enter first integer: ");
if (input.hasNextInt()) {
number1 = input.nextInt();
}
System.out.printf("You entered number %s \n", number1);
}
}
This will just print 0 if you try typing something that isn't an int. Once you learn about exceptions, you'll learn more ways to improve on this code. But this code you posted is just meant as a simple input / output example, so there's no need to overthink it.

garylacumbre
12,265 PointsYour code does run just fine on my computer with TexMate so your explanation is probably right. I think I will stick with Workspaces for my assignments. Thanks again for taking the time to help me out!
nikhil davasam
2,182 Pointsnikhil davasam
2,182 Pointsi think this is becoz u have written print instead of printf in the 6th line hope it worked!