Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial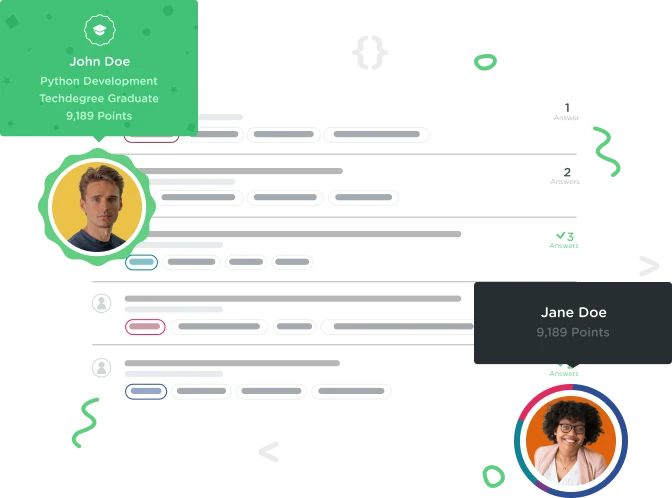

Destiny Robbins
210 PointsJava console bank app
So I wanted to write a basic console based banking app to practice my skills. It makes use of BigDecimal to ensure accuracy. Here is the MVP I plan to enhance it with all banking features soon. I tried to follow the MVC idea as close as possible, I'm pretty happy with the outcome, although I was questioning myself about some things like how I was creating the new account. As of now it can only accept one user, I plan to add some sort of data persistence like SQLite to practice with. Also there is no check to enforce the user to enter their details when creating an account so those fields can be blank. I will be enhancing this in the near future. Any peer reviews greatly appreciated on how my style could be improved, better way of handling how I did it or anything etc.
Main.java
public class Main
{
public static void main(String[] args)
{
Prompter prompter = new Prompter();
prompter.greetUser();
do
{
prompter.prompt();
prompter.processPrompt();
} while(!prompter.isFinishedBanking);
}
}
Prompter.java
import java.util.Scanner;
public class Prompter
{
protected boolean isFinishedBanking;
protected boolean areCustomerDetailsCorrect;
private String option;
private Scanner scanner = new Scanner(System.in);
private Account account = new Account();
public void prompt()
{
isFinishedBanking = false;
System.out.print("> ");
option = scanner.nextLine();
}
public void processPrompt()
{
if (option.toLowerCase().equals("exit"))
{
System.out.println("\nThank you for banking with JBank, goodbye!");
isFinishedBanking = true;
}
else if (option.toLowerCase().equals("home"))
{
System.out.println();
greetUser();
}
else if (option.toLowerCase().equals("options"))
{
showOptions();
}
else if (option.toLowerCase().equals("open"))
{
if(account.isUserCustomer)
{
System.out.println("Error! you have already opened an account.");
}
else
{
do
{
openAccountPrompt();
System.out.println("\nAre these details correct? Y/n");
printCustomerDetails();
prompt();
if (option.toLowerCase().equals("n"))
{
areCustomerDetailsCorrect = false;
}
else
{
areCustomerDetailsCorrect = true;
account.openAccount();
System.out.println("\nCongratulations! You have successfully opened a new account.");
}
} while(!areCustomerDetailsCorrect);
}
}
else if (option.toLowerCase().equals("deposit"))
{
if (!account.isUserCustomer)
{
System.out.println("\nError! you must open an account before you can use this option.\n");
}
else
{
System.out.println("\nEnter amount you with to deposit.");
prompt();
account.depositFunds(option);
System.out.println("\nSuccess! your new balance is: " + account.getAccountBalance());
}
}
else if (option.toLowerCase().equals("check"))
{
if (!account.isUserCustomer)
{
System.out.println("\nError! you must open an account before you can use this option.\n");
}
System.out.println();
System.out.println("Your account balance is: " + account.getAccountBalance());
}
else if (option.toLowerCase().equals("withdraw"))
{
if (!account.isUserCustomer)
{
System.out.println("\nError! you must open an account before you can use this option.\n");
}
else
{
System.out.println("\nEnter amount you with to withdraw.");
prompt();
if (account.checkAccountForAvailableFunds(option))
{
System.out.println("\nError! you don't have the available funds in your account to complete this transaction. Your available balance is: " + account.getAccountBalance());
}
else
{
account.withdrawFunds(option);
System.out.println("\nSuccess! your new balance is: " + account.getAccountBalance());
}
}
}
else
{
System.out.println("\nError! I didn't recognize your response, please try again.\n");
}
}
public void openAccountPrompt()
{
System.out.println("\n\nEnter your first name.");
prompt();
account.setCustomerFirstName(option);
System.out.println("\nEnter your last name.");
prompt();
account.setCustomerLastName(option);
System.out.println("\nEnter your address.");
prompt();
account.setCustomerAddress(option);
System.out.println("\nEnter your phone number.");
prompt();
account.setCustomerPhoneNumber(option);
System.out.println("\nEnter your email address.");
prompt();
account.setCustomerEmailAddress(option);
System.out.println("\nEnter amount to fund your new account.");
prompt();
// If left blank defaults to zero.
if (option.equals(""))
{
option = "0";
}
account.setAccountBalance(option);
}
public void printCustomerDetails()
{
System.out.printf("Name: %s %s\nAddress: %s\nTelephone number: %s\nEmail address: %s\nBeginning balance: %s\n\n",
account.getCustomerFirstName(), account.getCustomerLastName(),
account.getCustomerAddress(),
account.getCustomerPhoneNumber(),
account.getCustomerEmailAddress(),
account.getAccountBalance());
}
public void greetUser()
{
System.out.println("Welcome to JBank! Type \"options\" for a list of options, \"home\" to get back here, or \"exit\" to exit.\n");
}
public void showOptions()
{
System.out.println("\nOptions: \"open\" an account, \"deposit\" funds, \"check\" balance, or make a \"withdraw\"\n");
}
}
Account.java
import java.math.BigDecimal;
public class Account
{
public boolean isUserCustomer = false;
private Account customerAccount;
private String customerFirstName;
private String customerLastName;
private String customerAddress;
private String customerPhoneNumber;
private String customerEmailAddress;
private String stringAccountBalance;
private BigDecimal bigDecimalAccountBalance = BigDecimal.ZERO;
public Account()
{
}
public Account(String customerFirstName, String customerLastName, String customerAddress, String customerPhoneNumber, String customerEmailAddress, String stringAccountBalance)
{
this.customerFirstName = customerFirstName;
this.customerLastName = customerLastName;
this.customerAddress = customerAddress;
this.customerPhoneNumber = customerPhoneNumber;
this.customerEmailAddress = customerEmailAddress;
this.stringAccountBalance = stringAccountBalance;
}
public String getCustomerFirstName()
{
return customerFirstName;
}
public void setCustomerFirstName(String customerFirstName)
{
this.customerFirstName = customerFirstName;
}
public String getCustomerLastName()
{
return customerLastName;
}
public void setCustomerLastName(String customerLastName)
{
this.customerLastName = customerLastName;
}
public String getCustomerAddress()
{
return customerAddress;
}
public void setCustomerAddress(String customerAddress)
{
this.customerAddress = customerAddress;
}
public String getCustomerPhoneNumber()
{
return customerPhoneNumber;
}
public void setCustomerPhoneNumber(String customerPhoneNumber)
{
this.customerPhoneNumber = customerPhoneNumber;
}
public String getCustomerEmailAddress()
{
return customerEmailAddress;
}
public void setCustomerEmailAddress(String customerEmailAddress)
{
this.customerEmailAddress = customerEmailAddress;
}
public String getAccountBalance()
{
return bigDecimalAccountBalance.toString();
}
public void setAccountBalance(String stringAccountBalance)
{
this.stringAccountBalance = stringAccountBalance;
bigDecimalAccountBalance = new BigDecimal(stringAccountBalance);
}
public void openAccount()
{
customerAccount = new Account(customerFirstName, customerLastName, customerAddress, customerPhoneNumber, customerEmailAddress, stringAccountBalance);
isUserCustomer = true;
}
public void depositFunds(String stringDepositAmount)
{
BigDecimal bigDecimalDepositAmount = new BigDecimal(stringDepositAmount);
bigDecimalAccountBalance = bigDecimalAccountBalance.add(bigDecimalDepositAmount);
}
public void withdrawFunds(String stringWithdrawAmount)
{
BigDecimal bigDecimalWithdrawAmount = new BigDecimal(stringWithdrawAmount);
bigDecimalAccountBalance = bigDecimalAccountBalance.subtract(bigDecimalWithdrawAmount);
}
public boolean checkAccountForAvailableFunds(String stringWithdrawAmount)
{
boolean isAvailable;
BigDecimal bigDecimalWithdrawAmount = new BigDecimal(stringWithdrawAmount);
// Checks to make sure there are enough funds in the account to make the withdraw.
if (bigDecimalAccountBalance.subtract(bigDecimalWithdrawAmount).compareTo(BigDecimal.ZERO) < 0)
{
isAvailable = true;
}
else
{
isAvailable = false;
}
return isAvailable;
}
}