Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial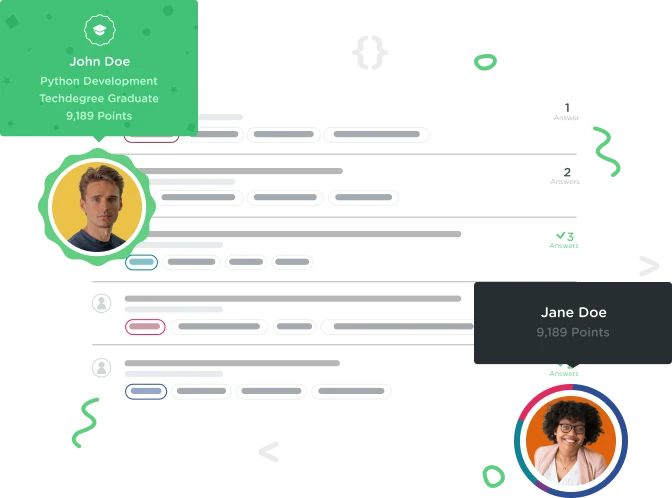
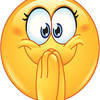
Bogdan Siverchuk
Courses Plus Student 1,707 PointsJava Data Structures. Challenge 1 of 2
i need to return obj as String if it is a String.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
return result;
}
}
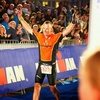
Steve Hunter
57,712 PointsThe obj
is passed into the method - it doesn't matter where it comes from - it's inside your method and called obj
- that's all we need to know.
7 Answers
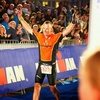
Steve Hunter
57,712 PointsHi there,
Use instanceof
to see if
the obj
is a String
. If it is, cast it to String
and return that.
Start with the if
. The condition is the instanceof
expression. Inside the if
return the cast obj
.
Let me know how you get on.
Steve.
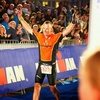
Steve Hunter
57,712 PointsI think of casting as in casting an actor to a character. So, you take a Will Smith and cast him as Robert Neville in I Am Legend. It converts the data type.
Anyway, for the last part of the challenge, cast to BlogPost
, then put more brackets all round that and use dot notation on the last bracket to call the getTitle()
method. That brings a string back, which you want to return.
You can either do that in the else
clause, or you can create a new if
statement to test using insdtanceof
. That's unnecessary, though as we know that obj
is either a String
or a BlogPost
. So, if it isn't a string, it's a blog.
I hope that helps.
Steve.
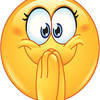
Bogdan Siverchuk
Courses Plus Student 1,707 Pointsincompatible types: Object cannot be converted to String
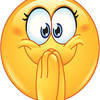
Bogdan Siverchuk
Courses Plus Student 1,707 Pointsi just wrote
if ( obj instanceof String){
return obj;
}
But the video says that i can fix it with the name of the class. String obj = (name_of_the_class) obj
??
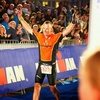
Steve Hunter
57,712 PointsYes! So put String
in brackets before obj
in your return
line.
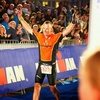
Steve Hunter
57,712 PointsOK - that's fine - but cast obj
when you return
it. Put (String)
before obj
in your return
line.
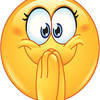
Bogdan Siverchuk
Courses Plus Student 1,707 PointsWhy i put (String) and i can't put just String(i tryed and failed)
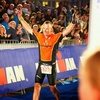
Steve Hunter
57,712 PointsThat's the way the language works - to cast to a type, the brackets are needed. When you declare something, they're not needed. Have you put (String)
in your return line?
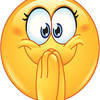
Bogdan Siverchuk
Courses Plus Student 1,707 PointsYes. It worked, Thank you for that, but i just wanted to know why i put brakets. Thx again
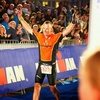
Steve Hunter
57,712 PointsIt's just one of those things - that's how casting works. No idea why it's needed technically!
Bogdan Siverchuk
Courses Plus Student 1,707 PointsBogdan Siverchuk
Courses Plus Student 1,707 PointsI don't understand where does he get this Object obj, and how i should make it String!