Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial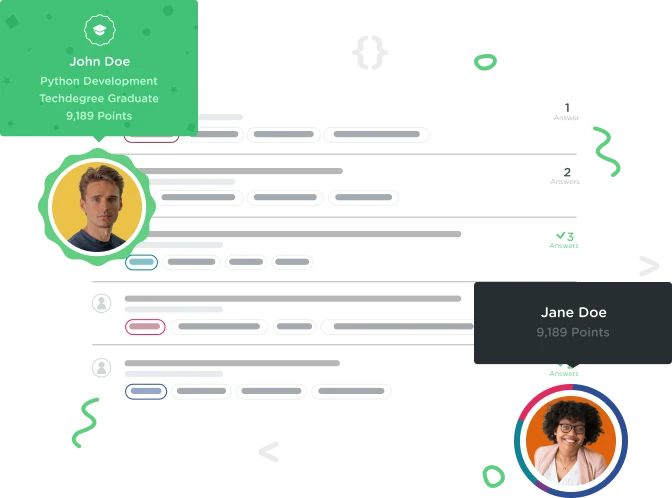
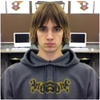
Ian Z
14,584 PointsJava Data Structures challenge comparable uses if(equals()) how does this work
In this step, cast the Object passed to the compareTo method to a com.example.BlogPost. Check for equality first, if they are equal return 0. Leave the default return of 1 in place for this step.
the code is
@Override
public int compareTo(Object obj){
BlogPost bp = (BlogPost)obj;
if(equals(bp)){
return 0;
}
return 1;
}
dosent equals(bp) need to be called on something? what is equals comparing bp to?

Ryan Jin
15,337 PointsYou use this.equals, like this
public int compareTo(Object obj){
if (this.equals(obj)){
obj = (BlogPost)obj;
return 0;
}
return 1;
}
8 Answers
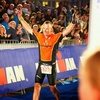
Steve Hunter
57,712 PointsHi Ian,
Have a look at this post which might help you figure out what's required.
I've got as far as:
public int compareTo(Object obj){
if(obj instanceof BlogPost){
BlogPost bp = (BlogPost) obj;
return 0;
}
return 1;
}
Steve.
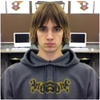
Ian Z
14,584 PointsHey Steve, thanks for help, i wasnt very clear on this sorry, i just linked code because it had example of equals() without a . before it and id never seen that before and was wondering what it was called on
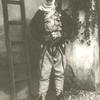
amathiaitishar
13,229 PointsThe java course is full of strange challenges, it never stops surprising me ...Why?!!!
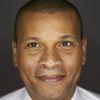
Neil Gordon
8,823 Pointsthis is what worked for me on the challenge
public int compareTo(Object obj) {
if(equals(obj)) {
return 0;
}
return 1;
}

Rulon Taylor
2,762 PointsHi Neil,
Doesn't the code you posted simply compare obj to obj? Thus, it should always evaluate as true and return 0. Right?
I'm thinking the code needs to be more like
BlogPost bp = (BlogPost) obj if(equals(obj)) { return 0; } return 1; }
What am I missing?

Dawid Dlubek
1,583 PointsThis worked:
public int compareTo(Object obj) { BlogPost post = (BlogPost) obj; if (equals(post)) { return 0; } return 1; }

Craig Dennis
Treehouse Teacherequals
is a method also inherited from java.lang.Object
. All Objects have an equals
method, so this is basically comparing the instance to the passed in value, in this case the BlogPost
instance bp
.
That make sense?
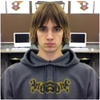
Ian Z
14,584 PointsYes thanks very much, so its kinda like this.equals

kristoffer tølbøll
1,337 PointsMakes no sense...
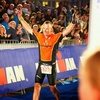
Steve Hunter
57,712 PointsHi Kristoffer,
What are you having problems with?
Steve.

kristoffer tølbøll
1,337 PointsHello Steve, i was not trying to be rude. Sorry if i sounded that way. I read in the javadoc, athat if you implement the comprable class you also have to put the class name in <>.
like this
public Class A implements comparable <A>
i did not see this syntax in the challenge i'm i missing something?
i Also have a hard time understanding the compareTo method could you elaborate
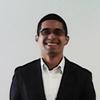
Darwin Palma
3,430 PointsIt worked for me in the challenge.
public int compareTo(Object obj){
if(equals(obj)){
BlogPost blogPost = (BlogPost)obj;
return 0;
}
return 1;
}

Mike Stanton
Courses Plus Student 3,250 Points//Create a new method overriding the existing class method 'compareTo' @Override public int compareTo(Object obj){ //Create a new BlogPost instance object (newBlogPost) for Comparing: BlogPost newBlogPost = (BlogPost) obj; //Here is where we are casting the object passed into the method to a BlogPost object. //Check for equality of those two Objects: if(equals(obj){ //This is saying: if the class Object BlogPost == the casted Object obj return 0. In this case, because we cast the object to a BlogPost object, then we should get 0 return 0; } return 1; }
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHave you got the link to that challenge? I agree with you that it looks odd like that.
Steve.