Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial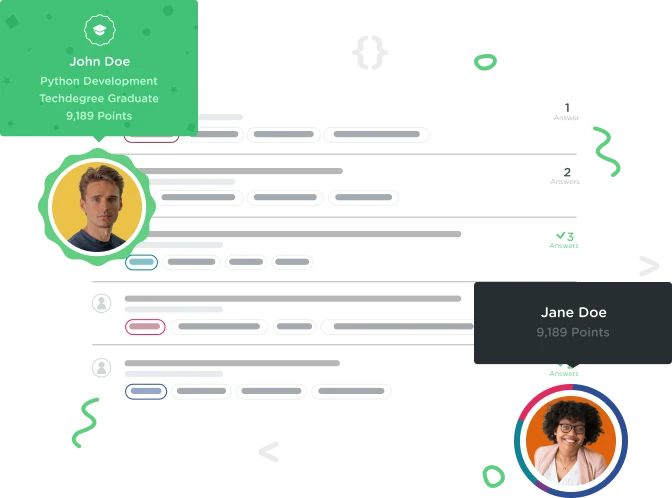
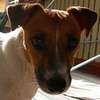
Robert Mylne
13,708 PointsJava Error!
I am getting this error with my code: Bummer! Something went very wrong. java.lang.IllegalAccessException: Class JavaTester can not access a member of class com.example.BlogPost with modifiers ""
Not sure what I am doing wrong though
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
BlogPost(String author, String title, String body, String category, Date creationDate)
{
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
}
3 Answers
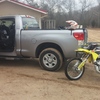
Ryan Ruscett
23,309 PointsHey,
You are super close.
java.lang.IllegalAccessException
The error refers to access modifiers, which are things like "public", "private", "protected". The next part of the error "can not access a member of class com.example.BlogPost with modifiers" is saying that the class BlogPost which includes the class and the constructor. Can't be accessed because their are issues with the modifiers.
public BlogPost (String author, String title, String body, String category, Date creationDate)
Just add public to your BlogPost constructor. And you are all set. This will work. A constructor always needs to have an access modifier. You are saying the class is public, so the constructor is public too. Or else JavaTester can't access the class (Class + constructor) if that class is not public including the constructor.
Does this make sense? I can explain it more since this is a high level overview of it. Just let me know if this works for you and or if you have further questions.
Thanks!!
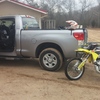
Ryan Ruscett
23,309 PointsHey,
When you do not define a constructor. Java automatically creates a no arg default constructor. The default constructor is given the access rights as the class. So if the class is private, so is the default constructor. If the class is public than so is the default constructor. Since not every class needs information upon being created. You don't need to define a constructor in every class.
Let me ask you this.
- Did anyone ever say JavaTester was in the same package as BlogPost? JavaTester is the class trying to access your BlogPost class.
Now by that logic, Your class is public, your constructor should be public by default. This seems like you would still be right, at least that would make sense... BUT lol, always a BUT!
When you call a constructor and pass in arguments to that constructor. That means it's NOT a default constructor. It means that the compiler now knows, that this class can never be instantiated unless it has it receives the required args. A default constructor ONLY has no-args. Once you pass in args, it's no longer a default and an access modifier is required. To put it simply. Now this can change if you have a super class or not, or even multiple constructors. Which we don't in this case.
So the take home is, always define your constructors. I see people get tripped up by this all the time when using inner classes or nesting classes. When other who wrote the class didn't define the modifiers properly. Inheritance of access modifiers from the class are ok for methods no matter what the args are, but for constructors it matters since it's what sets up the class on creation.
Does that help or have I confused you more?
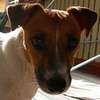
Robert Mylne
13,708 PointsA bit of both haha. I do understand what you are saying though.
No constructor: Default constructor is use with package visibility 'default' Constructor defined with no visibility: The constructor can't take the visibility of the class like methods as it hasn't been instantiated yet?
Question: then if it hasn't been defined what visibility does it take?
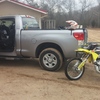
Ryan Ruscett
23,309 PointsA class can have a constructor defined, or you can have a class without defining the constructor. If the class doesn't define any constructor at all. The compiler creates one. This is called the default constructor. This default constructor is also known as a no args constructor. Since it doesn't require anything in order to set up the class when it's instantiated. These constructors take on the access modifier associated with the class. If the class is public the default constructor is. If the class is private, than so is the default constructor.
Now, when you have a constructor that takes arguments. This tells the compiler that in order to instantiate this class, it needs some additional information. It relies on some other class to pass this data in. If I have a constructor that takes arguments. But I don't put an access modifier on it. It will default to package level visibility.
In the case of this exercise. The javaTester class is in a different package. Which means you are required to put an access modifier on the constructor. Because the constructor is being called by a method outside the package.
There isn't or at least I can't think of a good reason to ever not put an access modifier on a method and or constructor. Despite the fact that it has a default modifier, doesn't mean it's a good idea to let java use it. Unless you are the only one to ever work on your code, and it's not complex. I guess you could let java do the work. But I wouldn't recommend it.
Does that help?
Robert Mylne
13,708 PointsRobert Mylne
13,708 PointsHi, thanks :)
Great explanation.
I heard though from someone that if no visibility is declared it defaults to 'default' visibility, which I believe is package level. If this is correct shouldn't it have still worked?