Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial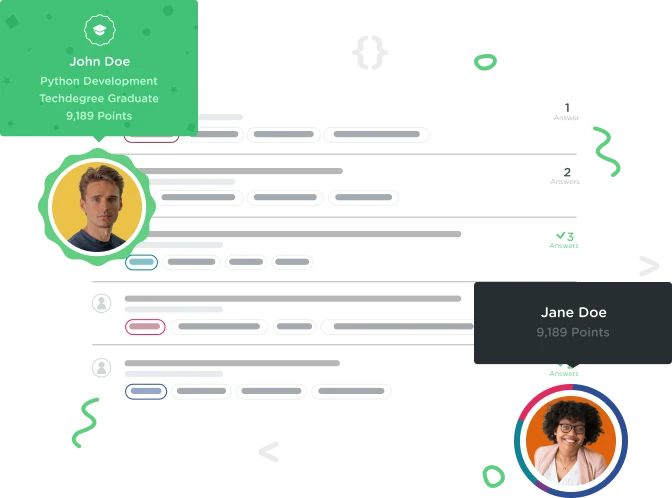
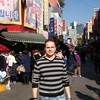
David Rojas
1,510 PointsJava error: missing return statement?
I really do not understand how to get around this error, I have read already lots of examples about the error but I am kind of lost!
I do not know what is missing or wrong.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 1;
}
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
3 Answers

Unsubscribed User
3,801 PointsConsider the following block of code:
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 1;
}
}
You have an if
statement, but no else
, and no code after this if
statement. If equals(other)
returns false
, your code will not be able to return an int
value, which is why the compiler is telling you that you are missing a return statement.
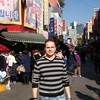
David Rojas
1,510 PointsThank you, now it is clear to me!

howardlim2
1,014 Pointsmissing return statement because it is possible that the object are not equal then no return statement

Alain Perez
Courses Plus Student 32 Pointspublic class NewTester {
public static String main(String[] args) {
int num=252,sumFirst=0,sumLast=0;
String first,last;
String value = String.valueOf(num);
if ( value.length() % 2 ==0) { //checks for even values
first =value.substring(0,value.length()/2-1);//works for even
last =value.substring(value.length()/2+1,value.length());//works for even
for(int i = 0; i < first.length(); i++) {
int j = Character.digit(first.charAt(i), 10);//returns each digit in string as int
sumFirst += j;}
for(int i = 0; i < last.length(); i++) {
int j = Character.digit(last.charAt(i), 10);//returns each digit in string as int
sumLast += j;}
if ( sumFirst == sumLast )
{
return "Balanced";
}}
else
return "Not Balanced";
if ( value.length() % 2 !=0)
{
first =value.substring(0,value.length()/2);//works for odd
last =value.substring(value.length()/2+1,value.length());//works for odd
for(int i = 0; i < first.length(); i++) {
int j = Character.digit(first.charAt(i), 10);//returns each digit in string as int
sumFirst += j;}
for(int i = 0; i < last.length(); i++) {
int j = Character.digit(last.charAt(i), 10);//returns each digit in string as int
sumLast += j;}
if ( sumFirst == sumLast )
{
return "Balanced";
}}
return "Not Balanced";
}
}
Alain Perez
Courses Plus Student 32 PointsAlain Perez
Courses Plus Student 32 PointsMissing return statemnt.