Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial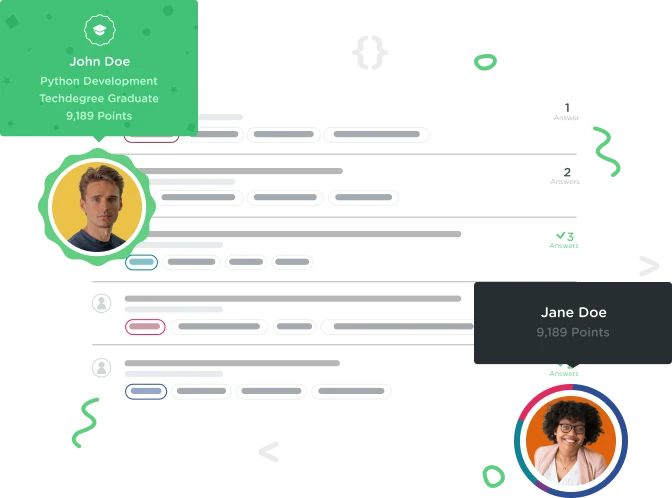

laurensvanhaecke
Courses Plus Student 899 PointsJava Help
Using Java.
Write class, Publisher, with attributes name and state.
Write Book class with attributes, title, author, isbn, publisher, and price. Book class has method calculateCharge that accepts the quantity sold and returns the total charge.
Write two children of Book: Fiction and NonFiction. Fiction has attribute fiction code. NonFiction has attribute category code.
Data will be in a two-dimensional data array as follows:
Fiction, Abraham Lincoln Vampire Hunter, Grahame-Smith, Wiley, NY, 978-0446563079, 13.99, 222
Fiction, Frankenstein, Shelley, Prescott, GA, 978-0486282114, 7.99, 321
NonFiction, Life of Kennedy, Jones, Pearson, MT, 758-29304566, 12.90, biography
Fiction, Dracula, Stoker, Addison, CA, 978-0486411095, 5.99, 145
Fiction, Curse of the Wolfman, Hageman, Wesley, MA, B00381AKHG, 10.59, 876
NonFiction, How to Pass Java, Willis, Wiley, NY, 444-395869790, 1.99, technology
Fiction, The Mummy, Rice, Addision, CA, 978-0345369949, 7.99, 954
NonFiction, History of Texas, Smith, Prescott, CA, 123-683947687, 9.75, history
- Rewrite the test case to create all books and calculate the charge and print out as before.
The quantity will be in a one-dimensional array as follows.
12 8 3 53 7 23 14 5 6.
Using the data array, create instances of books and store them in a one-dimensional array of Book, bookArray. Using the quantity array, walk through the book array and calculate the total charge per book sold. Print in a dialog box the book title, the book isbn, and the total charge for each book. Then, print the grand total of all books sold.
1 Answer
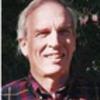
jcorum
71,830 PointsLaurens,
The first problem is that the order of the fields in your constructors is not the same as the order of the fields in your data. You have fictionCode and categoryCode as the first fields in Fiction and NonFiction constructors, but the last fields in your data. So you need to put them last in the constructors:
public Fiction(String title, String author, Publisher publisher, String isbn, double price, String fictioncode)
{
super(title, author, publisher, isbn, price);
setFictioncode(fictioncode);
}
public NonFiction(String title, String author, Publisher publisher, String isbn, double price, String categorycode)
{
super(title, author, publisher, isbn, price);
setCategorycode(categorycode);
}
The second issue is that for Fiction you have defined fictionCode as a String, your constructor expects a String, and your data has it as a String, but you were trying to turn it into an int using parseInt() in BookTest when you constructor a new Fiction. So I replaced Integer.parseInt(dataArray[i][7]) with dataArray[i][7]. If you really want to make it an int you will need to change your data, the type of the private instance variable, your constructor, etc.
The third problem is the way you are trying to create Publisher objects in BookTest. Here's an alternative that will compile:
for (int i = 0; i < bookArray.length; i++)
{
if (dataArray[i][0].equals("Fiction"))
{
Publisher p = new Publisher(dataArray[i][3], dataArray[i][4]);
bookArray[i] = new Fiction(dataArray[i][1], dataArray[i][2],
p, dataArray[i][5], Double.parseDouble(dataArray[i][6]), dataArray[i][7]);
}
else
{
Publisher p = new Publisher(dataArray[i][3], dataArray[i][4]);
bookArray[i] = new NonFiction(dataArray[i][1], dataArray[i][2],
p, dataArray[i][5], Double.parseDouble(dataArray[i][6]), dataArray[i][7]);
}
}
What this does is construct the publisher objects first, and then it uses that constructed object in the constructors for Fiction and NonFiction.
With these changes you code will now compile.
laurensvanhaecke
Courses Plus Student 899 Pointslaurensvanhaecke
Courses Plus Student 899 PointsThis is what I have so far.
public class Publisher { private String name; private String state;
}
public class Book { private String title; private String author; private Publisher publisher; private String isbn; private double price;
}
public class Fiction extends Book { private String fictioncode;
}
public class NonFiction extends Book { private String categorycode;
}
import javax.swing.JOptionPane;
public class BookTest { public static void main(String args[]) { String dataArray [][] = {{"Fiction", "AbrahamLincolnVampireHunter","Grahame-Smith","Wiley", "NY", "978-0446563079", "13.99", "222"}, {"Fiction", "Frankenstein", "Shelley", "Prescott", "GA", "978-0486282114", "7.99","321"}, {"NonFiction", "LifeofKennedy","Jones","Pearson","MT","758-29304566","12.90","biography"}, {"Fiction","Dracula","Stoker","Addison","CA","978-0486411095","5.99","145"}, {"Fiction","CurseoftheWolfman","Hageman","Wesley","MA","B00381AKHG","10.59","876"}, {"NonFiction","HowtoPassJava","Willis","Wiley","NY","444-395869790","1.99","technology"}, {"Fiction","TheMummy","Rice","Addison","CA","978-0345369949","7.99","954"}, {"NonFiction","HistoryofTexas","Smith","Prescott","CA","123-683947687","9.75","history"}};
}