Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial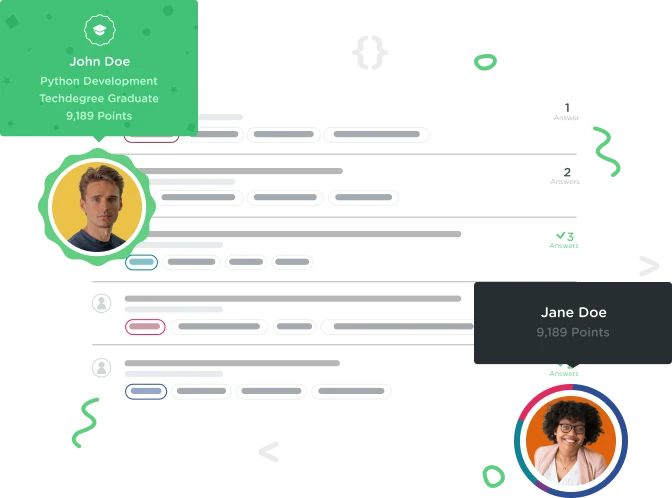

Steven Bell
660 PointsJava Help
Write a program that reads a four-digit integer, such as 1998, and then displays it, one digit per line, like so: 1 9 9 8 Your prompt should tell the user to enter a four-digit integer. You can then assume that the user follows directions. (Hint: Use the division and remainder operators.)
3 Answers
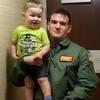
Taylor Hyles
Courses Plus Student 782 Pointsimport java.util.*;
import java.io.Console;
public class FourDigit {
public static void main(String[] args) {
Console console = System.console();
LinkedList<Integer> stack = new LinkedList<Integer>();
int digits = 0;
do {
String response = console.readLine("Enter a four digit integer:");
try{
digits = Integer.parseInt(response);
}
catch (NumberFormatException e){
}
if (1000 > digits || digits > 9999){
System.out.println("Please try again...");
}
}
while(1000 > digits || digits > 9999);
while (digits > 0) {
stack.push( digits % 10 );
digits = digits / 10;
}
while (!stack.isEmpty()) {
System.out.println(stack.pop());
}
}
}
Hopefully this is good enough, added a couple of things for if the user mistypes or tries to break the program...

Steven Bell
660 PointsHi Simon, I think I mad an oops! The output of the four-integers entered should be display as: 1/n, 9/n, 9/n, 8/n. Each number should print on a new line. Sorry.
Steve

Simon Coates
28,694 Pointsthe system.out.println methods i've been using print to individual lines. And you can add \n to strings eg. System.out.print("leading up to a new line.\nI am on a new line"); when using something that doesn't automatically do the new lines.
and fyi, the forward slash is usually used to imply division. backslash is used with escape chars. Treehouse has a markdown syntax if you want to display output or code (otherwise, it gets weird on white space)
1
9
9
8

Steven Bell
660 PointsThanks! So, I will I approach the program? How can I make each number in the four-digits print on different lines after the user inputs a four-digit number?

Simon Coates
28,694 PointsThe program has two tasks. Get input in. I included how to get it using system.in, while there was Taylor demoed using console. Both of these included getting you an int. Then having a four digit number, then you either use a collection and a loop (taylor and I have given you some options) or go the really schlocky route, which i demoed with:
public static void main(String[] args) {
int digits = 1998; //assume 4 digits long
int firstDigit = digits % 10;
digits = digits / 10;
int secondDigit = digits % 10;
digits = digits / 10;
int thirdDigit = digits % 10;
digits = digits / 10;
int fourthDigit = digits % 10;
System.out.println(fourthDigit );
System.out.println(thirdDigit);
System.out.println(secondDigit );
System.out.println(firstDigit);
}
you just need to cut, paste and rename. Everything you need to do has been demoed here.
Simon Coates
28,694 PointsSimon Coates
28,694 PointsKeep in mind that if submitting this for an introductory java course, the LinkedList, and exception handling may not have been covered yet.
Steven Bell
660 PointsSteven Bell
660 PointsThis is a introductory class and a most of the handling in this program has not been covered yet. Is there another way to achieve the same outcome without the advance steps? Thanks in advance, Steve
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsif you haven't covered exception handling, you just strip that out. A lot of introductory courses just assume that you're entering valid inputs. And how you get inputs in may differ depending on environment. The introductory java courses i took at uni, tended to get inputs from System.in. The following shows two examples of this.
Simon Coates
28,694 PointsSimon Coates
28,694 PointsThe basic ways to store a collection might be an array (requires a fixed length), or an ArrayList. Here's some arraylist code:
or an array demo:
Steven Bell
660 PointsSteven Bell
660 PointsThanks for your help but this is too advance for an introductory class.
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsstill too advanced? I guess you can bypass using an array or arraylist, if you don't use a loop. maybe something like
but this is really, really basic.