Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial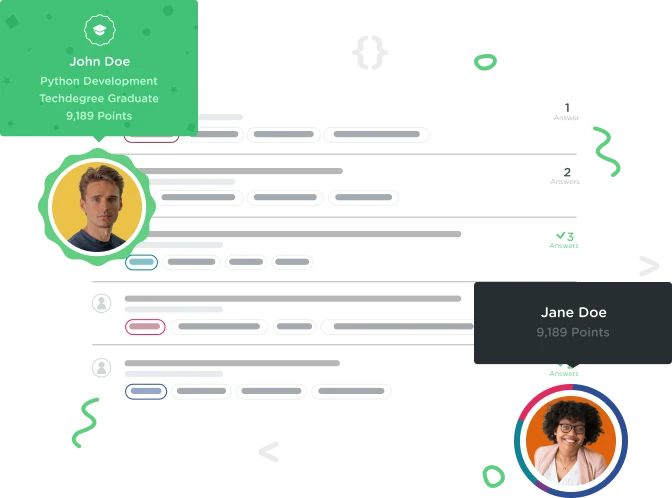

Michelle Bramlett
1,253 PointsJava Helper Method Challenge Task 2 receiving 1 error Ln 26 "Cannot find symbol" Stuck! Please help
Been stuck on this challenge too long:/ Researched other answers on forum and still not passing Insight, please!
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
if (isBatteryEmpty()) {
mBarsCount ++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
if(!isBatteryEmpty) {
return true;
}
}
}
1 Answer

Simon Coates
28,694 Pointsgot it to work with:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
public boolean isBatteryEmpty(){
return mBarsCount == 0;
}
public boolean isFullyCharged(){
return mBarsCount == MAX_BARS;
}
}

Michelle Bramlett
1,253 PointsThanks Simon! My code was getting way to complicated as I was trying to get help. I can follow the simplicity of yours above( and it worked;)
Simon Coates
28,694 PointsSimon Coates
28,694 PointsThe following still crashes, but shows the updated code.
I get the message "Bummer! Your method should return true when mBarsCount is full.". However, it goes away when I copy in:
which has the original charge method. SO YOU DID CHANGE SOMETHING IN ADDITION TO THE TASK AND IT MAY HAVE BEEN GIVING YOU FAULTY ERROR MESSAGES.