Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial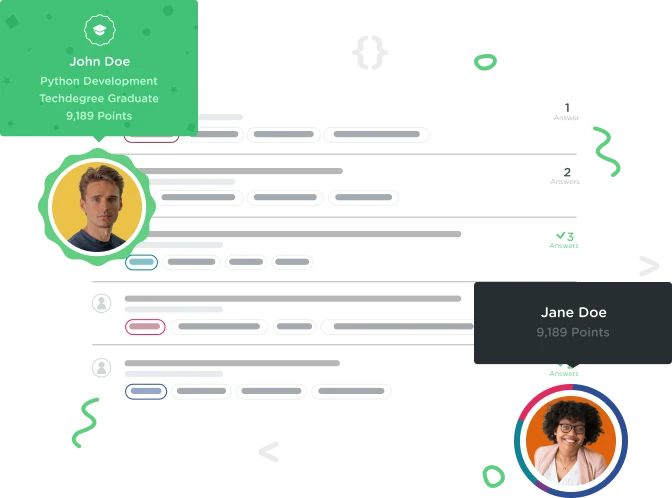

Björn Norén
9,569 PointsJava Multiply big numbers Question
Hi! If I multiply 300 * 1000 * 1000 * 1000 in Java I get the number: -647710720 Could someone explain why it isn't 300 000 000 000?
I use cmd.exe
Here's the code:
public class Test
{
public static void main (String[] args)
{
System.out.println(300 * 1000 * 1000 * 1000);
}
}
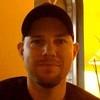
Jeremy Hill
29,567 PointsLike this:
public class Test{
public static void main (String[] args)
{
long num = 300L * 1000L * 1000L * 1000L;
System.out.println(num);
}
}
3 Answers

Ken Alger
Treehouse TeacherBjorn;
That is a great question.
In looking at some of the limitations on number types Java has, you will see that the int
data type is a 32-bit signed two's complement integer. It has a maximum value of 2,147,483,647(inclusive), (2^31 -1).
For your code above, you will want to utilize a data type which allows for a larger range. If you use the long
data type it is a 64-bit signed two's complement integer. It has a maximum value of 9,223,372,036,854,775,807 (inclusive), (2^63 -1) and used by designating the number as a Long type with the L
character. You can add that to one of the numbers in your equation and the entire number will be converted to a Long type. For example:
public class Test
{
public static void main (String[] args)
{
System.out.println(300 * 1000 * 1000 * 1000L);
}
}
Post back with further questions.
Happy coding, Ken

Björn Norén
9,569 PointsHi Ken, thanks for reaching out and helping me, I appreciate it alot!
I apologize, I should have emphazied that I wonder WHY it results in such a random number, and that I know that it should be utilized to a long, for example. So, is this a way for Java to say that the number -647710720 equals 300 000 000 000, when defualted to a double? Is that accurate?
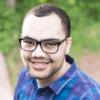
Philip Gales
15,193 PointsIt is defaulting to a double, which is why it always shows that random number. Try explicitly telling it that it is a long.
//long x = 300L * 1000L * 1000L * 1000L;
long x = 300 * 1000 * 1000 * 1000;
System.out.println(x);
You may notice I have 2 lines with 1 commented out. Even though I have assigned both to a long, without the "L" telling java each number is a long it will still multiply as if it were a double.
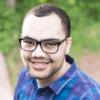
Philip Gales
15,193 PointsFor anything large like 50 digit or more you can use a BigInteger.

Björn Norén
9,569 PointsHi everyone, thanks for reaching out and helping me, I appreciate it alot!
I apologize, I should have emphazied that I wonder WHY it results in such a random number, and that I know that it should be utilized to a long, for example. So, is this a way for Java to say that the number -647710720 equals 300 000 000 000, when defualted to a double? Is that accurate?
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsI don't know why it gave that output but I do know that you should use type Long for big numbers.