Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial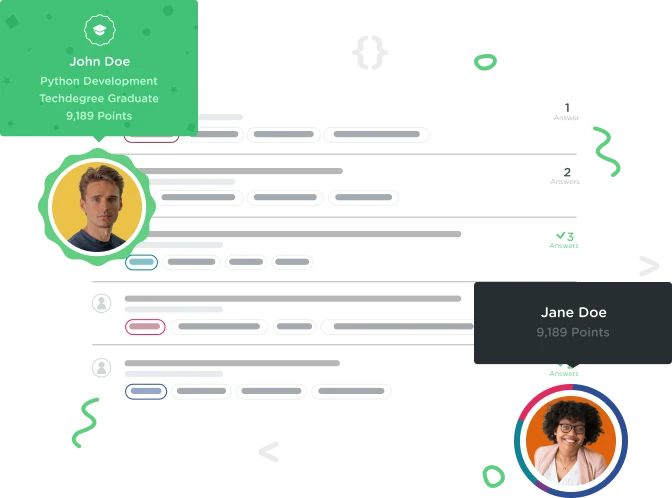

Derek Derek
8,744 PointsJava: Multiply without using * or /
Hello, Treehouse community!
I am trying to implement a way to multiply two numbers without using * or /. Below is a code from a source, and I need some help understanding how it works. The part I don't understand is
int halfProd = multiply(s, bigger);
Could you please tell me what s and halfProd are at each time of recursive step and also how you got those numbers? Thank you so much for reading this and helping me out!
public class Multiply {
public static void main(String[] args) {
System.out.println(multiply(8, 11));
}
public static int multiply(int a, int b) {
int smaller = a < b ? a : b;
int bigger = a < b ? b : a;
if (smaller == 0) {
return 0;
}
else if (smaller == 1) {
return bigger;
}
int s = smaller >> 1;
int halfProd = multiply(s, bigger);
if (smaller % 2 == 0) {
return halfProd + halfProd;
}
else {
return halfProd + halfProd + bigger;
}
}
}
Simon Coates
28,694 PointsSimon Coates
28,694 PointsIf you want to look at values in general, run the program with breakpoints and step through it, or add System.out.println statements. Here you might want to add indenting to communicate the depth of recursion of the values. I think the algorithm you're using is actually standard for integer division, hence you should be able to find a decent explanation online.
Using 8 and 11: The smaller argument is shifted, while the larger argument in unaltered. 8 (00001000), becomes 4(00000100), 2, and then 1. the recursion with 1 returns 11. The recursion with 2 returns 22. The recursion with the smaller number of 4 returns 44. the initial method call with smaller number of 8 returns 88.