Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial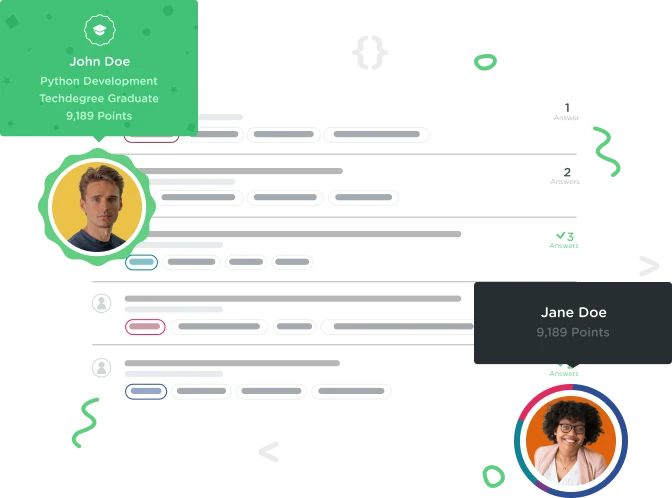
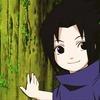
Matthew Francis
6,967 Points[Java] Newbie - I don't think I'm invoking this method properly
public class HelloWorld{
public static void main(String []args){
System.out.println("Hello World");
Toys nToy = new Toys();
nToy.Dog();
}
}
public class Toys{
public String lol;
public String Dog(){
lol = "Hi world";
System.out.printf("%s", lol);
}
}
--
any ideas what's wrong?
2 Answers

Benjamin Barslev Nielsen
18,958 PointsYou are invoking the method correctly, but there are a couple of problems with the implementation. Do you have the two classes in separate files or are they in one file? You can only have one public class in each file. If they are in the same file, you can skip the public keyword on Toys, but I would suggest multiple files, when you have these two classes.
About the method:
public String Dog(){
lol = "Hi world";
System.out.printf("%s", lol);
}
You specify it returns a String, but you do not return anything. System.out.printf's writing is a side-effect, not something that is returned. Therefore you should either change the return type to void, or return a String. And please don't make methods start with a capital letter, only constructors should start with a capital letter in Java.

Abhinav Kanoria
7,730 PointsFirst, you should put different classes in separate folders and import the packages that you need (it's recommended). Secondly, you specified the return type of Dog() function to be a String, yet you are not returning a String. Change the return type to void.
Matthew Francis
6,967 PointsMatthew Francis
6,967 PointsI have them on separate files, but what is the downside of having them in the same file?
Totally forgot about that! just for future references, is there any way to return a value without the return keyword?
Benjamin Barslev Nielsen
18,958 PointsBenjamin Barslev Nielsen
18,958 PointsDownside to having them in the same file:
In large projects it becomes harder to maintain the code, if you just have some huge files with a lot of classes that has different responsibilities. For instance I would never look inside the HelloWorld file to find the Toys class, so if my collaborator had done this, I would spend a lot of time just figuring out how to find the Toys class.
"is there any way to return a value without the return keyword":
Short answer: No, the only way to return is through the return keyword. However using side-effects the results of a function might still be available to you, even though the return type is void, this is for instance the case with the sort method on array. We might expect it to return a sorted array, but it doesn't return anything, instead it has modified the array you gave as input.