Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial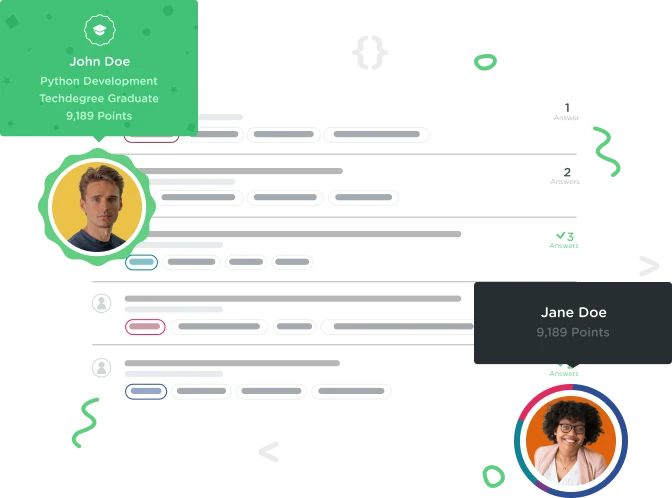

Tashfin Shahid
1,298 Points(Java) Not sure what the syntax error is?
Not sure what the syntax error is in this part of the code based on the question below
if (lastName.charAt(0) == 'a','b','c','d','e','f','g','h','i','j','k','l','m')
{
lineNumber +=1;
}
else{
lineNumber +=2;
}
return lineNumber;
}
I've created a tool to help manage lines at tech conferences. The organizers would like to split the attendees into two lines during the registration process. I've added notes, examples and a new lesson in the Example.java tab.
Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
if (lastName.charAt(0) == 'a','b','c','d','e','f','g','h','i','j','k','l','m')
{
lineNumber +=1;
}
else{
lineNumber +=2;
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer

Lauren Moineau
9,483 PointsYou can only assign a single value to a char. Here, you're checking it against a list of different values, as if writing:
if (x == 10, 5, 3, 6, 15) // which is not correct
One way would be to check the char against each value:
if (lastName.charAt(0) == 'A' || lastName.charAt(0) == 'B' || lastName.charAt(0) == 'C' ... )
but there is an easier way since we can compare chars, as we do numbers. So just like if you wanted to check if a number x was between 10 and 150 (incl) you would write: if (x >= 10 && x <=150), you can do the same with letters, checking if a char c is between A and M:
if (c >= 'A' && c <= 'M')
// Use capital letters here as the comparison is case sensitive and last names start with a capital letter
The second point to be careful about is that you should not increment the value of the variable lineNumber, but rather set its value to either 1 or 2, accordingly. So, wrapping it all up, you get:
if (lastName.charAt(0) >= 'A' && lastName.charAt(0) <='M') {
lineNumber = 1;
} else {
lineNumber = 2;
}
return lineNumber;
}
Hope this helps
Tashfin Shahid
1,298 PointsTashfin Shahid
1,298 PointsThis website in my experience has the best community. In StackOverflow I find it's not welcoming for beginners based on my experience when asking questions whereas over here people like you in the community really do go the extra mile not to just give an answer, but to explain the thought process whilst providing constructive criticism for how I approached my own solution. Thank you very much for taking your own time to explain this very clearly :)
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsThank you very much for your comment. I'm glad I could be of any help :) I agree SlackOverflow is very intimidating and not beginner-friendly when it comes to asking questions. I was lucky to have the help of some student communities when I started so, although I'm still no expert, I'm happy to now be the one to lend a hand whenever I can :)