Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial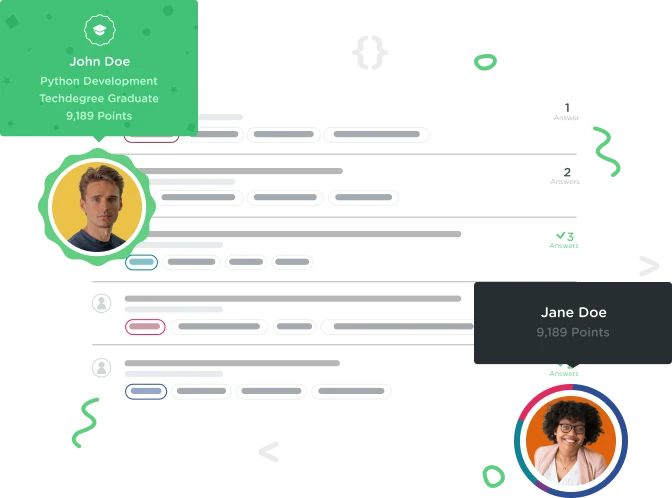

John Hay
Courses Plus Student 3,478 PointsJava Object: Meet Objects
Hi, I didn't really get any of this session although was able to pass the challenges. Should I redo it all again in the hope that it will make more sense or should I just carry on in the hope that these concepts will be covered again. Not sure how to best approach this.
1 Answer

Matthew Caloger
12,903 PointsObjects in Java are an extremely important concept. While you can continue on and see if you'll get it down, maybe a different explanation would help.
in Object-Oriented languages like Java, "classes" are used as a mold for creating variables with a specified set of properties and functions attached.
For example, say you're writing a program that needs to keep track of contact information, you may have a class that looks like the following:
public class Contact {
// properties
String firstName;
String lastName;
String phoneNumber;
// constructor
public Contact (String initialFirstNameValue, String initialLastNameValue, String initialPhoneNumberValue) {
this.firstName = initialFirstNameValue;
this.lastName = initialLastNameValue;
this.phoneNumber = initialPhoneNumberValue
}
// methods
public String getFirstName() {
return this.firstName
};
public String setFirstName(String newFirstName) {
this.firstName = newFirstName;
};
public String getLastName() {
return this.lastName
};
public String setLastName(String newLastName) {
this.lastName = newLastName;
};
public String getPhoneNumber() {
return this.phoneNumber
};
public String setPhoneNumber(String newPhoneNumber) {
this.phoneNumber = newPhoneNumber;
};
}
The important parts here are the properties of the class, the constructor, and the methods.
In our example, each Contact
would have a first name, last name, and phone number we could assign. The constructor would allow us to set initial values for those names, and then we have getter and setter functions to retrieve or change that information. Every time we declare a new Contact variable, we'd need to set those values, and then we can access them using the methods we created.
Objects are complex, and there's a lot of depth to them that you'll learn about, but pretend it's a contract you're making with the object to have the given properties and methods available to them, and they need whatever the constructor is to get started.