Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial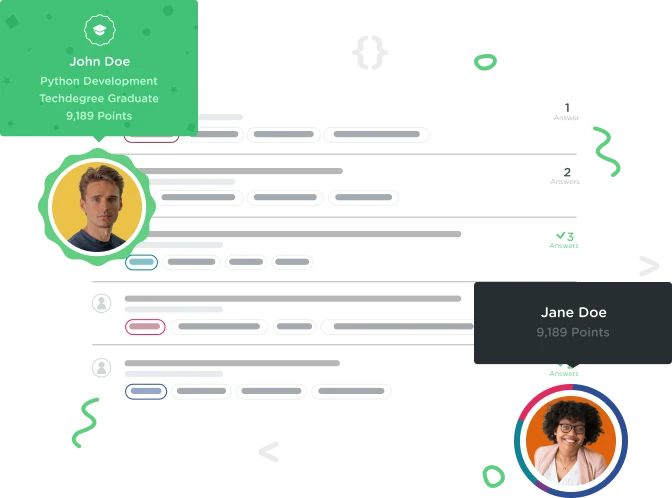
3 Answers
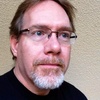
Chris Freeman
Treehouse Moderator 68,423 PointsIt may help to think of an object as a instantiation of a class. Functions or methods defined within the class can be be associated with the class (class functions) or with the object instantiated from the class (instance functions). When programming, a class method is signified with the word "static".
How I approach the solution is to consider the general form for a method, then fill it in based on the task description.
// general method structure
<access> <returns> <name>(<arguments>) {
<action code>
return <result>
}
For the challenge, you are asked to create two helper methods. Let's build it by filling in the sections above based on the task description:
// Task 1 of 2
// Create a helper method that returns whether or not the GoKart needs
// to be charged (<action>)
// This is asking for a True or False result so return a boolean <(returns)>
// Make it public (<access>) and name it isBatteryEmpty (<name>).
// so:
// access is "public"
// returns is "boolean"
// name is "isBatteryEmpty()"
// arguments is None
// action code is "GoKart needs charging"
// This is determined by "mBarsCount == 0"
// result is boolean value
public boolean isBatteryEmpty() {
// combining the return result and action code
return mBarsCount == 0;
}
// Task 2 of 2
// Now create a helper method named isFullyCharged (<name>). It should
// return true (<returns>) if the GoKart is at max capacity (<action code>).
// so:
// access is not specified so assume "public"
// returns is "boolean"
// name is "isFullyCharged()"
// arguments is None
// action code is "GoKart is at max capacity"
// This is determined by "mBarsCount == MAX_BARS"
//
public boolean isFullyCharged() {
// combining the return result and action code
return mBarsCount == MAX_BARS;
}

Tony K
Courses Plus Student 442 PointsVery Nice Explanation Chris Freeman

jinhwa yoo
10,042 Pointsperferct... I understand everything.. now.. omg...
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsGreat explanation, Chris !!!!