Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial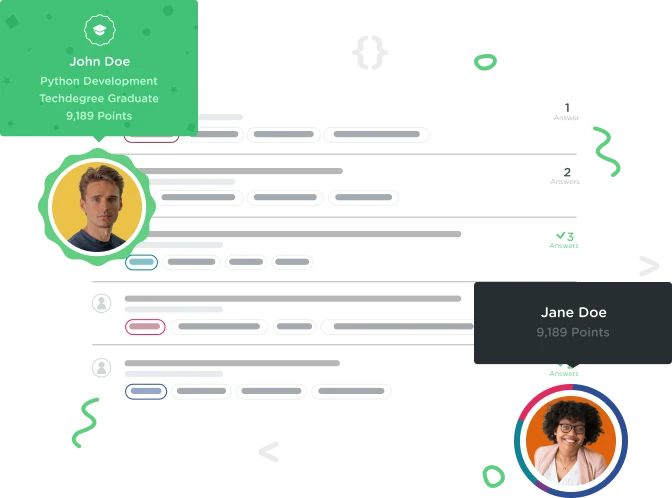

Quinn Manning
3,045 PointsJava Objects - Applying a Discount Code - 2 of 2
The task given to me is to normalize the discountCode string so that it may only contain letters and the char '$'.
This is for task 2 of 2, the first tasks only requirement was to create a normalizeDiscountCode method and have it return discountCode in upper case which I completed very easily.
Now when I run the below code for task 2 of 2 it doesnt throw any errors and I can only assume it fulfilled the requirements, however the message I get back is that the requirements for task 1 are no longer being fulfilled, and I dont see how that can be the case.
From what I can see is that for every character in string discountCode it is checked agains the acceptable values and if there are no problems then it returns string discountCode.toUpperCase(). Why is this not reading the returned string as upper case?
Thanks for your time!
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
normalizeDiscountCode(discountCode);
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode (String discountCode) {
for (char c : discountCode.toCharArray()) {
if (! Character.isLetter(c) || c != '$') {
throw new IllegalArgumentException("Invalid Discount Code");
}
}
return discountCode.toUpperCase();
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHi Quinn
This part of your code is the main source of problem:
private String normalizeDiscountCode (String discountCode) {
for (char c : discountCode.toCharArray()) {
if (! Character.isLetter(c) || c != '$') {
throw new IllegalArgumentException("Invalid Discount Code");
}
}
return discountCode.toUpperCase();
The main problem here is in your IF statement. Basically you want to say that the discountCode can only consist of letters or '$' character. Thus if not you should throw exception. Yes logically you should say IF it's NOT( a letter OR '$') then throw exception. Therefore the IF statement should be like:
if (! (Character.isLetter(c) || c = '$'))
Or if you still want to distribute the negation logical operator ('!') you must change your IF statement to be (NOT letter AND NOT '$') like this:
if (! Character.isLetter(c) && c != '$')
For further reasoning on why the logical operator should be arranged like that you can Google it to learn more. I hope this will help you a little.

Quinn Manning
3,045 PointsThank you so much, that makes perfect sense. That was going to legitimately drive me insane!
Simon Coates
28,694 PointsSimon Coates
28,694 PointsI think what Yanuar Prakoso is referring to is called 'De Morgan's laws' (if you want to google for full explanation).
! (A || B) is !A && !B.
!(A && B) becomes !A || !B