Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial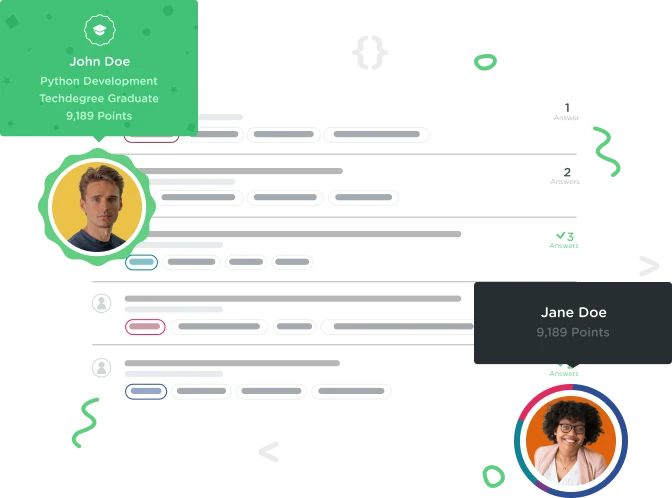
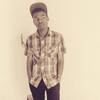
MUZ140584 Heredity Mbundire
4,979 Pointsjava objects delivering the mvp challenge 1
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
Boolean startsWithLetterM = fieldName.startsWith("m");
Boolean secondLetterIsUppercase = Character.isUpperCase(fieldName.charAt(1));
if (!startsWithLetterM || !secondLetterIsUppercase) {
throw new IllegalArgumentException();
}
help
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User(args[0],args[1]);
// Add the author, title and description
ForumPost post = new ForumPost(author, "Wow at Last", "Java is Awesome");
forum.addPost(post);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
5 Answers
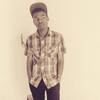
MUZ140584 Heredity Mbundire
4,979 Pointsthanks i ddnt c that am confused
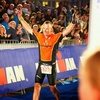
Steve Hunter
57,712 PointsIt's a lot of code for one challenge, but the amount you have to write is minimal.
You need to create a new addItem
method that only takes one parameter, the Product item
bit. It doesn't need a quantity
parameter as, in this scenario, that is set to 1.
The existing code takes two parameters:
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
You need to override that and create the same method with just one parameter:
public void addItem(Product item) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
So, I deleted the second parameter from the method - but will the rest of the code work? No. The printf
will fail because it requires a quantity
. However, we know that if there's no quantity
specified, this means the user wants a single item. So replace quantity
with the number 1, as an integer.
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", 1, item.getName());
/* Other code omitted for clarity */
}
Then you need to uncomment the call to this modified function in the Example
class.
cart.addItem(dispenser);
That should do it for you. If the user passes in two parameters, the function will return quantity
number of item
. If the user only sends in the item
they'll get a single one.
I hope that makes sense.
Steve.
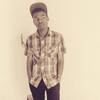
MUZ140584 Heredity Mbundire
4,979 Pointsam stil stuck this is my code
public class Example {
public class ShoppingCart {
public void addItem(Product item, int quantity) {
quantity = 1;
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
// cart.addItem(dispenser);
}
}
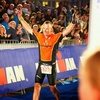
Steve Hunter
57,712 PointsHiya,
You've mixed the code from the Product
class into Example
. You only need to make a couple of amendments in this challenge.
I'll copy the code into here so you can see what I mean.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
/**
You uncomment the line below - it is commented out in the challenge
**/
cart.addItem(dispenser);
}
}
So, you are making a call to the addItem
function above but with only one parameter. You need to add another version of the addItem
method that will take a single parameter. The code below does that - the explanation is in my earlier reply.
You don't need to change any other code.
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product item) {
System.out.printf("Adding %d of %s to the cart.%n", 1, item.getName());
/* Other code omitted for clarity */
}
}
This code stays exactly the same as at the start of the challenge - you change nothing in here:
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
So those are the complete, correct three files that will pass the challenge. If you have changed a lot, refresh the page to get back to the beginning and start again. Or delete each file in turn and copy this code into each one.
Steve.

Jess Sanders
12,086 PointsYour best bet is to start the challenge over.
Example.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are Adding 1 of the item to the cart.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi!
The code you have posted doesn't all relate to this challenge, I don't think.
The
Example.java
should be different to what you have posted. And the listing forTeacherAssistant
isn't required, I don't think.This challenge requires you to amend the
addItem
method so that you can just pass it a singleproduct
name and no quantity to buy one of the item. The existing solution requires a number to be passed, even if it is 1.I hope that makes sense - best check you code so you're working with the right lists.
Steve.