Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial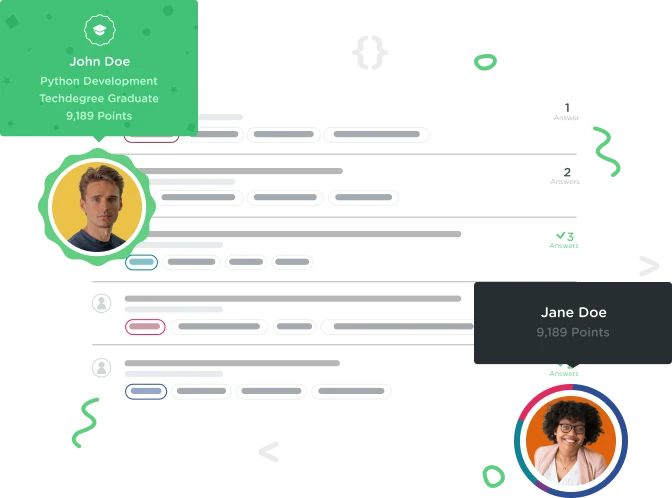

djwyatt
13,954 PointsJava Objects / Methods and Constants Challenge Task 1 of 3
These GoKarts have a single rechargeable battery that have a display of bars to measure its energy level. Each battery has a maximum of 8 bars.
For this task, let's add a constant field to the class that stores the maximum number of energy bars. Make sure the field cannot be changed and is accessible from the class, not just the instance. Use the naming convention we learned.
public class GoKart {
private String mColor;
public GoKart(String color) {
mColor = color;
}
public String getColor() {
return mColor;
public class Bars {
public static final int MAX_BARS = 8;
private String mCharacterName;
private int mBarsCount;
}
}
}
3 Answers
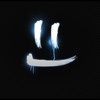
Grigorij Schleifer
10,365 PointsHi dywyatt,
allow me to comment this challenge ....
public class GoKart {
private String mColor;
public static final int MAX_BARS = 8;
// I deleted "public class Bars" because we only need to define variables here
// public means the variable is accessible from the class, and not just the instance (so correct)
// final: it cannot be changed (also correct)
//TASK 2
private int mBarsCount;
// an uninitialized int variable
// constructor has the same name as the class and it needs to be public
// so you can use/access the constructor from everywhere
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
// initializing means assigning a value to a variable
// here we initialize the value 0 to mBarsCount
}
// a public method
// that returns nothing (void)
// a method need the word void, not like the constructor
public void charge () {
mBarsCount = MAX_BARS;
// assigning MAX_BARS (= 8) to the mBarsCount variable
// when you call charge() you set mBarsCount from 0 to 8 !
}
public String getColor() {
return mColor;
}
}
Does it make sense?
Grigorij

djwyatt
13,954 PointsThanks for the help, yes it does make sense! Much appreciated.

Gavin Ralston
28,770 PointsAll you need to do in this case is add MAX_BARS as a static, final field to the GoKart class. :)
You don't need to make a Bars subclass to hold on to the constant or anything. Just put it right there in the GoKart class as a static field.
public class GoKart {
private String mColor;
public static final int MAX_BARS = 8;
public GoKart(String color) {
mColor = color;
}
public String getColor() {
return mColor;
}
}
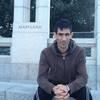
Muhammad Asif
Courses Plus Student 557 Pointspublic class GoKart { private String color; public static final int MAX_BARS = 8;
public GoKart(String color) { this.color = color; }
public String getColor() { return color;
} }
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey dywyatt
You are very welcome !!!
See you in the forum ...