Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial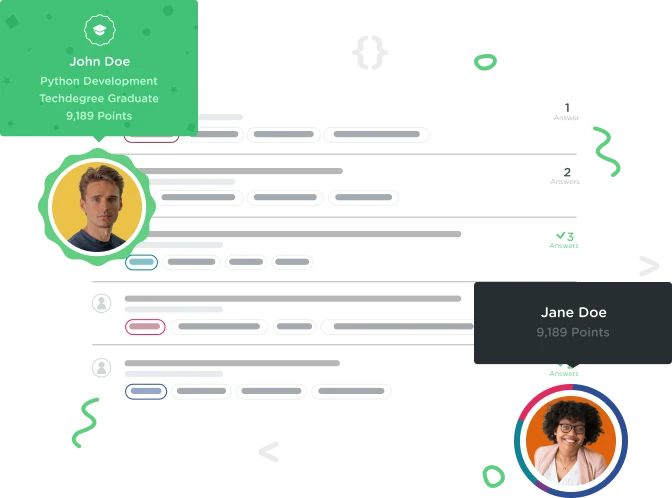

William J. Terrell
17,403 PointsJava Objects - Wrapping Up - Task 4 of 4
I still don't know how I made it through this course... I'm assuming the problem here is that the code for ForumPost in Example.java needs to be similar to the code for User author?
My code is below; see the comment in Example.java, line 12.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
public String getDescription() {
return mDescription;
}
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public void setFirstName(String firstName) {
mFirstName = firstName;
}
public void setLastName(String lastName) {
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
//When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
//Take the first two elements passed args
User author = new User(args[0], args[1]);
// Add the author, title and description
ForumPost post = new ForumPost(/*something has to go here, right?*/);
forum.addPost(post);
}
}
So far, for the ForumPost post = new ForumPost(-something here-), I've tried args, User, String, etc. All present errors...
Any assistance is appreciated.
Thanks!
3 Answers

William J. Terrell
17,403 PointsAha! I got it! You just pass in "author", plus what you want it to say. (I'm not going to post exactly what I actually put in, but...)
ForumPost post = new ForumPost(author, "I'm", "Done!");

Craig Dennis
Treehouse TeacherYou made it through because you are awesome!

William J. Terrell
17,403 PointsSo you are here :) I wonder why it didn't notify me that you had left a response. That's one of the many things I like about Treehouse, is that the teachers are actually "there" (or "here"...somewhere...available...respondent...alive...you get the idea...).
I left another post that I was wondering if you would have anything to say about, visible here:
https://teamtreehouse.com/forum/java-data-structures-2
Also, just to better understand how the comments section works, if I use Craig Dennis (typing in @craigdennis and then clicking on the name from the list that appears), will it notify you directly?
Thanks!
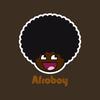
adsdev
15,583 Pointsi finished this challenge, but iยดm a little curious as to why and which effect it has when modifying the code to the first to arguments.
/Take the first two elements passed args User author = new User(args[0], args[1]);
Can someone elaborate this as to what part the args[0], args[1] refer to ??