Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial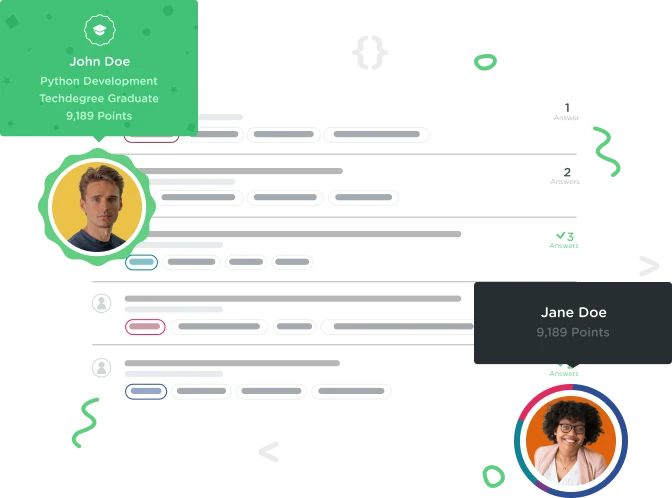

Derek Derek
8,744 Points[Java] permutation with duplicates
I am following Cracking The Coding Interview section 8.8: permutation with duplicates. But it seems that my outcome is incorrect. Could you spot where the bug is?
Also, in the recursion part of the printPerms method, after the recursive line, what does map.put(c, count); do? I thought that we need to decrement the count for each character until it becomes zero, so I don't get why we restore the count.
import java.util.Arrays;
import java.util.ArrayList;
import java.util.HashMap;
public class PermWithDups {
public static void main(String[] args) {
printPerms("Hello");
}
static ArrayList<String> printPerms(String s) {
ArrayList<String> result = new ArrayList<String>();
HashMap<Character, Integer> map = buildFreqTable(s);
printPerms(map, "H", s.length() - 1, result);
System.out.println(Arrays.toString(result.toArray()));
return result;
}
static void printPerms(HashMap<Character, Integer> map, String prefix,
int remaining, ArrayList<String> result) {
// Base Case for Recursion
if (remaining == 0) {
result.add(prefix);
return;
}
// Recursion
for (Character c : map.keySet()) {
int count = map.get(c);
if (count > 0) {
map.put(c, count - 1);
printPerms(map, prefix + c, remaining - 1, result);
map.put(c, count);
}
}
}
static HashMap<Character, Integer> buildFreqTable(String s) {
HashMap<Character, Integer> map = new HashMap<Character, Integer>();
for (char c : s.toCharArray()) {
if (!map.containsKey(c)) {
map.put(c, 0);
}
map.put(c, map.get(c) + 1);
}
return map;
}
}
1 Answer

Seth Kroger
56,413 PointsI don't know if you're suppose to find all permutations of the letters or only the permutations starting with 'H', but the issue is where you start the top call of the recursion. Either you need to start with in empty string or you forgot remove the starting letter(s) from the frequency table.
It looks like map.put(c, count); is there to unwind the changes to the frequency table as the recursion backs out so the level above it can move on to the next character choice. Otherwise, if you only decremented you'd only get one permutation and the rest would be skipped over.