Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial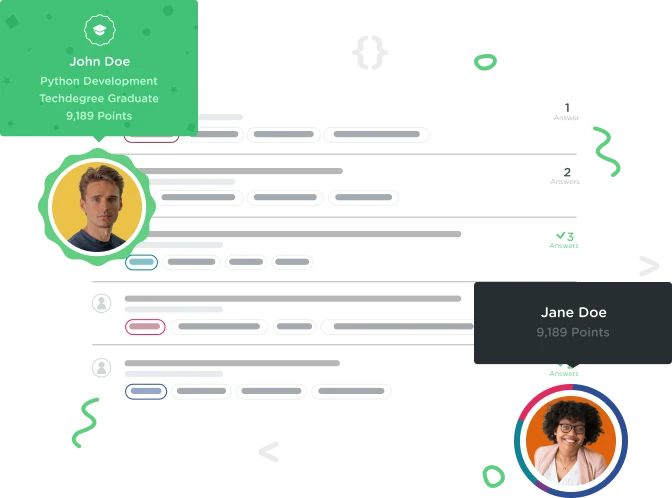
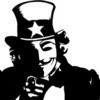
behar
10,799 PointsJava, printing a getter returns a weird value??
Hey guys! Im trying to build a simple TTT game in Java, but i noticed something weird. In a class i create an array like this:
public class Game {
private int[] board = {0, 1, 2, 3, 4, 5, 6, 7, 8};
public int[] getBoard() {
return board;
}
}
Then in my main file i try to do this:
public class Main {
public static void main(String[] args) {
Game game = new Game();
int[] test = game.getBoard();
System.out.println(test);
}
}
Now i excpected to get back {0, 1, 2, 3, 4, 5, 6, 7, 8}, but instead i get "[I@5f150435". Is that like a memory location or something, and if so, how would i print the actual array? Ty in advance!
1 Answer

andren
28,558 PointsNow i excpected to get back {0, 1, 2, 3, 4, 5, 6, 7, 8}, but instead i get "[I@5f150435". Is that like a memory location or something, and if so, how would i print the actual array? Ty in advance!
It's actually mainly the array's hashCode. It gets printed because the println
method automatically calls the array's toString
method. toString
is a method that all objects in Java have and that by default simply prints the class name of an object and its hashCode. A lot of classes override this method so that it returns a more useful String
representation of the object, but that is not done for array objects.
There are various ways of printing out the array, the simplest is likely to use the Arrays.toString
method. In order use this method you have to import java.util.Arrays
into your program.
Here is a code example:
import java.util.Arrays; // Import the class
class Main {
public static void main(String[] args) {
Game game = new Game();
int[] test = game.getBoard();
System.out.println(Arrays.toString(test)); // Call the toString method on the array
}
}
That will print out:
[0, 1, 2, 3, 4, 5, 6, 7, 8]
If you don't want to import anything then the simplest option would likely be to use a foreach loop. Like this:
class Main {
public static void main(String[] args) {
Game game = new Game();
int[] test = game.getBoard();
for (int item : test) { // Create a loop that goes through the array
System.out.println(item); // Print out each item of the array on a separate line
}
}
}
That will print out this:
0
1
2
3
4
5
6
7
8
If you wanted to print out the numbers on one line you could replace the println
method with the print
method.
behar
10,799 Pointsbehar
10,799 PointsTy so much! Excellent explanation!