Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial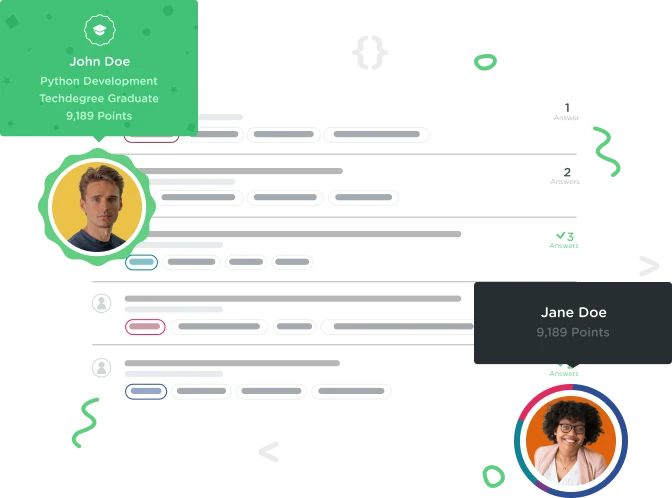
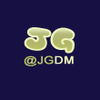
Jonathan Grieve
Treehouse Moderator 91,253 PointsJava Set Collection: My Review
I'm almost done these but if it helps others to understand what's going on then they'll have been worthwhile. I believe they're helping me :)
Sets are the collection interface that represents a list of unique values. It can take a list with repeated values and take out repeated data. A list that pulls in unique values.
A hash is a way to uniquely identify objects.
In Java every object has a method called hashCode() which is a 32-bit signed integer which can be overridden.
-
Several implementatons of Sets exist in Java including.
- Set
- SortedSet
- TreeSet
- HashSet
Set<String> (set of strings) nameOfSet = new HashSet<String>
- Sets and Lists can interact. You can quickly change the implementation of one Collection to another.
Set<String> uniqueLanguages = new HashSet<String>(allLanguages);
removes duplicate entries in a given list.
Set<String> uniqueLanguages = new TreeSet<String>(allLanguages); e.g. Hashsets can become TreeSets which sort automatically.
Requires import of java.util.Set and java.util.HashSet
Hashes. A Hash is a way to uniquely identify objects.
Set<String> - set of strings
Set<String> names = new HashSet<String>();
//add values to a set. The set retains only unique names.
names.add("James");
names.add("James");
names.add("Matthew");
names.add("Mark");
names.add("Matthew");
names.add("Mark");
names.add("Luke");
names.add("John");
//retrieve Set.
names;
//Add more names as an ArrayList.
List<String> names = Arrays.asList("Jon","Garfield","Odie");
Set<String> uniqueNames = new TreetSet<String>(names);
- SortedSets are an upgrade of TreeSets so more methods can be performed on them. such as headSet(), tailset() and subset();
SortedSet<String> uniqueNames = new TreeSet<String>(names);
set.headSet("J");
//returns everything beginning before J
set.tailSet("J");
//returns everything beginning after J
set.subSet("Java", "Java" + Character.MAX_VALUE);
//a searched list of everything beginning with J
HashSet - an implementation of a list with unique values only. TreeSet - an alphabetically sorted implementation of a list with unique values only.