Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial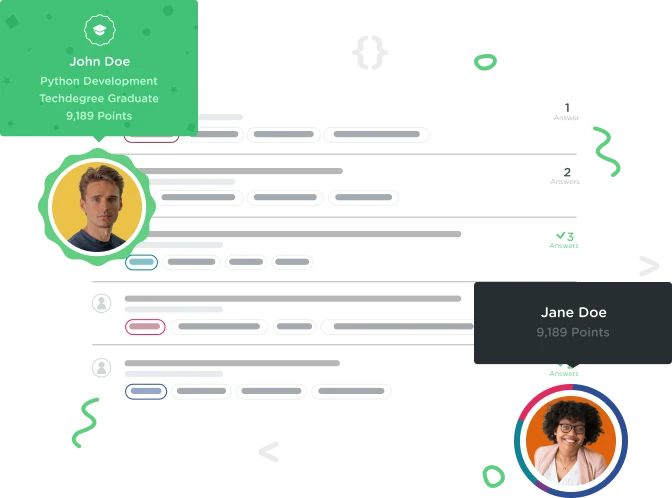

Conner Olsen
Courses Plus Student 1,670 PointsJava Sets
Ok so I've been busy and stopped doing treehouse for a while I just started doing it again and the first exercise I get lots of different errors. Could someone please tell me what I am doing wrong.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.list;
import java.util.set;
import java.util.*;
public class Blog {
List<BlogPost> mPosts;
public set<String> AllAuthors = new treeSet<String>();
AllAuthors.addAll(BlogPost.getAuthors());
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public getAllAuthors List<authors> {
return Allauthors
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Conner
You need to define the getAllAuthors method as public Set<String> getAllAuthors() because you will create a Set not a List of Strings. Set prohibits you to append two identical Stings in one Set. Then since the challenge requires you to sort the authors alphabetically you need to use TreeSet. Please do not forget to import both java.util.Set and java.util.TreeSet
I am attaching my code that I used to solve the particular challenge for your reference:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
//here is the answer:
public Set<String> getAllAuthors(){
Set<String> authors = new TreeSet<String>();
for(BlogPost post : mPosts){
authors.add(post.getAuthor());
}
return authors;
}
}
As you can see in the part of the code inside getAllAuthors() method I use for loops to extract each author from each blog post. Then I add it into the Set called authors. The Java will then make sure no identical or duplicate author name in the Set and since I am using TreeSet the Java program will ensure the author Names will be sorted alphabetically.
I hope this can help you a little.
Samuel Ferree
31,722 PointsSamuel Ferree
31,722 PointsYour code to initialize all your authors and add the posts to it is not syntactically valid, nor is it in the correct place
#2 is good advice for the initialization of AllAuthors as well. Let's look at how fixing these errors might look
These are just the errors I could see at a quick glance. If you post the exact errors, I can help you fix those as well.